Python: Remove a single entry in a list based on the position of the entry
Solution 1
These are methods you can try:
>>> my_list = ['hey', 'hi', 'hello', 'phil', 'zed', 'alpha']
>>> del my_list[0]
>>> my_list = ['hey', 'hi', 'hello', 'phil', 'zed', 'alpha']
>>> if 'hey' in my_list: # you're looking for this one I think
... del my_list[my_list.index('hey')]
...
>>> my_list
['hi', 'hello', 'phil', 'zed', 'alpha']
You can also use filter
:
my_list = filter(lambda x: x!='hey', my_list)
Using list comprehension
:
my_list = [ x for x in my_list if x!='hey']
Solution 2
First of all, never call something "list" since it clobbers the built-in type 'list'. Second of all, here is your answer:
>>> my_list = ['hey', 'hi', 'hello', 'phil', 'zed', 'alpha']
>>> del my_list[1]
>>> my_list
['hey', 'hello', 'phil', 'zed', 'alpha']
Solution 3
Lists work with positions, not keys (or names, whatever you want to call them).
If you need named access to your data structure consider using a dictionary instead which allows access to its value by using keys
which map to the values
.
d = {'hey':0, 'hi':0, 'hello':0, 'phil':0, 'zed':0, 'alpha':0}
del d['hey']
print(d) # d = {'alpha': 0, 'hello': 0, 'hi': 0, 'phil': 0, 'zed': 0}
Otherwise you will need to resort to index based deletion by getting the index of the element and calling del alist[index]
.
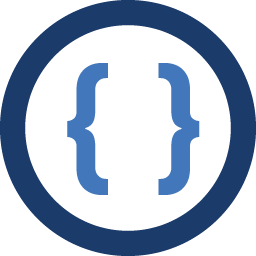
Admin
Updated on March 27, 2020Comments
-
Admin about 4 years
Is there an easy way to delete an entry in a list? I would like to only remove the first entry. In every forum that I have looked at, the only way that I can delete one entry is with the
list.remove()
function. This would be perfect, but I can only delete the entry if I know it's name.list = ['hey', 'hi', 'hello', 'phil', 'zed', 'alpha'] list.remove(0)
This doesn't work because you can only remove an entry based on it's name. I would have to run
list.remove('hey')
. I can't do this in this particular instance.If you require any additional information, ask.