Python-Requests close http connection
Solution 1
As discussed here, there really isn't such a thing as an HTTP connection and what httplib refers to as the HTTPConnection is really the underlying TCP connection which doesn't really know much about your requests at all. Requests abstracts that away and you won't ever see it.
The newest version of Requests does in fact keep the TCP connection alive after your request.. If you do want your TCP connections to close, you can just configure the requests to not use keep-alive.
s = requests.session()
s.config['keep_alive'] = False
Solution 2
I think a more reliable way of closing a connection is to tell the sever explicitly to close it in a way compliant with HTTP specification:
HTTP/1.1 defines the "close" connection option for the sender to signal that the connection will be closed after completion of the response. For example,
Connection: close
in either the request or the response header fields indicates that the connection SHOULD NOT be considered `persistent' (section 8.1) after the current request/response is complete.
The Connection: close
header is added to the actual request:
r = requests.post(url=url, data=body, headers={'Connection':'close'})
Solution 3
I came to this question looking to solve the "too many open files" error
, but I am using requests.session()
in my code. A few searches later and I came up with an answer on the Python Requests Documentation which suggests to use the with
block so that the session is closed even if there are unhandled exceptions:
with requests.Session() as s:
s.get('http://google.com')
If you're not using Session you can actually do the same thing: https://2.python-requests.org/en/master/user/advanced/#session-objects
with requests.get('http://httpbin.org/get', stream=True) as r:
# Do something
Solution 4
please use response.close()
to close to avoid "too many open files" error
for example:
r = requests.post("https://stream.twitter.com/1/statuses/filter.json", data={'track':toTrack}, auth=('username', 'passwd'))
....
r.close()
Solution 5
On Requests 1.X, the connection is available on the response object:
r = requests.post("https://stream.twitter.com/1/statuses/filter.json",
data={'track': toTrack}, auth=('username', 'passwd'))
r.connection.close()
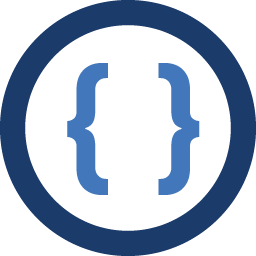
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I was wondering, how do you close a connection with Requests (python-requests.org)?
With
httplib
it'sHTTPConnection.close()
, but how do I do the same with Requests?Code:
r = requests.post("https://stream.twitter.com/1/statuses/filter.json", data={'track':toTrack}, auth=('username', 'passwd')) for line in r.iter_lines(): if line: self.mongo['db'].tweets.insert(json.loads(line))
-
Piotr Dobrogost about 12 yearsAlternatively
s = requests.session(config={'keep_alive': False})
-
btubbs over 11 yearsOP was asking how to close a streaming response. I don't see how setting keep-alive on the session is going to help there. There are several recent tickets in requests and urllib3 around this issue: github.com/shazow/urllib3/pull/132, github.com/kennethreitz/requests/issues/1073, github.com/kennethreitz/requests/issues/1041
-
Felix Fung over 11 yearsSee comment here: github.com/shazow/urllib3/pull/132#issuecomment-11516729
-
Martijn Pieters about 10 yearsCurrent
request
versions have no support for switching off Keep-Alive. -
Brad over 9 yearsWhat is 'body' in this case? just any { }?
-
Oleg Gryb over 9 years@Brad It depends on content-type, e.g. if you want to send application/json, you can use data=json.dumps(payload), if you just want to send a common form-encoded payload, you can use data={"k1":"v1", ...}. See more details here: docs.python-requests.org/en/latest/user/quickstart/…
-
gss almost 8 yearsAs of the current release you can ensure streaming connections go back to the pool by reading Response.content (or Response.text which calls Response.content). But if you are hoarding Response objects without reading them you will not be releasing your connections back: Documentation
-
Stefan Falk over 6 yearsThat's being used here but for some reason it appears that the connection is not really closed - I notices because my OS runs out of ports - which should not happen imho if that connection gets closed correctly. :/
-
dmigo over 5 yearsAm I supposed to downvote other comments in order to help this one to go up?
-
Ethan Furman over 4 years@dmigo: No. Only downvote if an answer is wrong in some way, or otherwise not useful to the question asked.
-
manoj prashant k about 4 yearsLets say i create a session using "with" in one function and return the session. Use the session in all my tests. How would i know if the session is terminated?
-
stwhite about 4 years@manojprashantk The best answer I can give is to test it in your code. Likely the request will be closed once you're out of the
with
block but you should read about Context Managers as it may have more of an answer to your question: docs.python.org/2/reference/datamodel.html#context-managers -
Melardev about 4 yearsAre you sure this works? requests.get() behind the scenes also uses the same construct, and the keep-alive is still working ...
-
Admin over 3 yearsTypeError: session() got an unexpected keyword argument 'config'
-
rohitcoder about 3 yearsI was looking for answers for exactly this problem!
-
Simplecode over 2 yearsWhat is the max. number of connections can a session handle before giving error "too many open files"