Python requests exception handling
Solution 1
Assuming you did import requests
, you want requests.ConnectionError
. ConnectionError
is an exception defined by requests
. See the API documentation here.
Thus the code should be :
try:
requests.get('http://www.google.com')
except requests.ConnectionError:
# handle the exception
Solution 2
As per the documentation, I have added the below points:-
-
In the event of a network problem (refused connection e.g internet issue), Requests will raise a ConnectionError exception.
try: requests.get('http://www.google.com') except requests.ConnectionError: # handle ConnectionError the exception
-
In the event of the rare invalid HTTP response, Requests will raise an HTTPError exception. Response.raise_for_status() will raise an HTTPError if the HTTP request returned an unsuccessful status code.
try: r = requests.get('http://www.google.com/nowhere') r.raise_for_status() except requests.exceptions.HTTPError as err: #handle the HTTPError request here
-
In the event of times out of request, a Timeout exception is raised.
You can tell Requests to stop waiting for a response after a given number of seconds, with a timeout arg.
requests.get('https://github.com/', timeout=0.001) # timeout is not a time limit on the entire response download; rather, # an exception is raised if the server has not issued a response for # timeout seconds
-
All exceptions that Requests explicitly raises inherit from requests.exceptions.RequestException. So a base handler can look like,
try: r = requests.get(url) except requests.exceptions.RequestException as e: # handle all the errors here
Solution 3
Actually, there are much more exceptions that requests.get()
can generate than just ConnectionError
. Here are some I've seen in production:
from requests import ReadTimeout, ConnectTimeout, HTTPError, Timeout, ConnectionError
try:
r = requests.get(url, timeout=6.0)
except (ConnectTimeout, HTTPError, ReadTimeout, Timeout, ConnectionError):
continue
Solution 4
for clarity, that is
except requests.ConnectionError:
NOT
import requests.ConnectionError
You can also catch a general exception (although this isn't recommended) with
except Exception:
Solution 5
Include the requests module using import requests
.
It is always good to implement exception handling. It does not only help to avoid unexpected exit of script but can also help to log errors and info notification. When using Python requests I prefer to catch exceptions like this:
try:
res = requests.get(adress,timeout=30)
except requests.ConnectionError as e:
print("OOPS!! Connection Error. Make sure you are connected to Internet. Technical Details given below.\n")
print(str(e))
continue
except requests.Timeout as e:
print("OOPS!! Timeout Error")
print(str(e))
continue
except requests.RequestException as e:
print("OOPS!! General Error")
print(str(e))
continue
except KeyboardInterrupt:
print("Someone closed the program")
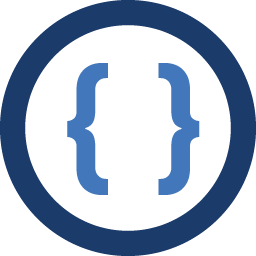
Admin
Updated on April 12, 2020Comments
-
Admin about 4 years
How to handle exceptions with python library requests? For example how to check is PC connected to internet?
When I try
try: requests.get('http://www.google.com') except ConnectionError: # handle the exception
it gives me error name
ConnectionError
is not defined