Python requests library how to pass Authorization header with single token
Solution 1
In python:
('<MY_TOKEN>')
is equivalent to
'<MY_TOKEN>'
And requests interprets
('TOK', '<MY_TOKEN>')
As you wanting requests to use Basic Authentication and craft an authorization header like so:
'VE9LOjxNWV9UT0tFTj4K'
Which is the base64 representation of 'TOK:<MY_TOKEN>'
To pass your own header you pass in a dictionary like so:
r = requests.get('<MY_URI>', headers={'Authorization': 'TOK:<MY_TOKEN>'})
Solution 2
I was looking for something similar and came across this. It looks like in the first option you mentioned
r = requests.get('<MY_URI>', auth=('<MY_TOKEN>'))
"auth" takes two parameters: username and password, so the actual statement should be
r=requests.get('<MY_URI>', auth=('<YOUR_USERNAME>', '<YOUR_PASSWORD>'))
In my case, there was no password, so I left the second parameter in auth field empty as shown below:
r=requests.get('<MY_URI', auth=('MY_USERNAME', ''))
Hope this helps somebody :)
Solution 3
This worked for me:
access_token = #yourAccessTokenHere#
result = requests.post(url,
headers={'Content-Type':'application/json',
'Authorization': 'Bearer {}'.format(access_token)})
Solution 4
You can also set headers for the entire session:
TOKEN = 'abcd0123'
HEADERS = {'Authorization': 'token {}'.format(TOKEN)}
with requests.Session() as s:
s.headers.update(HEADERS)
resp = s.get('http://example.com/')
Solution 5
i founded here, its ok with me for linkedin: https://auth0.com/docs/flows/guides/auth-code/call-api-auth-code so my code with with linkedin login here:
ref = 'https://api.linkedin.com/v2/me'
headers = {"content-type": "application/json; charset=UTF-8",'Authorization':'Bearer {}'.format(access_token)}
Linkedin_user_info = requests.get(ref1, headers=headers).json()
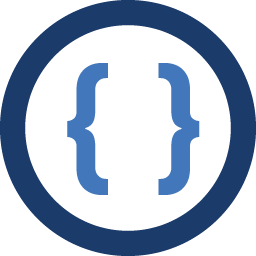
Admin
Updated on April 08, 2021Comments
-
Admin about 3 years
I have a request URI and a token. If I use:
curl -s "<MY_URI>" -H "Authorization: TOK:<MY_TOKEN>"
etc., I get a 200 and view the corresponding JSON data. So, I installed requests and when I attempt to access this resource I get a 403 probably because I do not know the correct syntax to pass that token. Can anyone help me figure it out? This is what I have:
import sys,socket import requests r = requests.get('<MY_URI>','<MY_TOKEN>') r. status_code
I already tried:
r = requests.get('<MY_URI>',auth=('<MY_TOKEN>')) r = requests.get('<MY_URI>',auth=('TOK','<MY_TOKEN>')) r = requests.get('<MY_URI>',headers=('Authorization: TOK:<MY_TOKEN>'))
But none of these work.
-
Admin over 10 yearsTraceback (most recent call last): File "<stdin>", line 1, in <module> File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/requests/api.py", line 55, in get return request('get', url, **kwargs) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/requests/api.py", line 44, in request return session.request(method=method, url=url, **kwargs) File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/requests/sessions.py", line 323, in request prep = self.prepare_request(req)
-
Ian Stapleton Cordasco over 10 years@rebHelium can you gist that? That is not the whole stack trace and there's no indication of what you actually tried.
-
Admin over 10 yearsSorry, Stack Overflow did not allow me to post the whole output. I did exactly as you suggested: r = requests.get('whatever url i have', headers={'Authorization': 'TOK:whatever token i have'})
-
Ian Stapleton Cordasco over 10 yearsNo need to apologize. Did it work? You accepted my answer but it seems to have caused an exception for you. If you create a gist I can help you with greater ease than having a conversation here.
-
Admin over 10 yearsSigma, I actually missed one small detail on your code that caused the error. The actual code is confidential so I cannot gist. But I will post additional questions since I want to improve my Python skills, if you want to look. They are very simple questions I am sure you would know.
-
Admin over 10 yearsThanks. Here's the link: stackoverflow.com/questions/19150208/… I haven't been able to use regex and get what I need. I will re-edit the question.
-
se_brandon over 7 yearsI was able to get it to work using: > r = requests.get('<MY_URI>', headers={'Authorization': 'MY_TOKEN_HERE'})
-
aydow over 6 yearsif you try
r = requests.get('<MY_URI>',auth=('<MY_TOKEN>'))
, you will getTypeError: 'str' object is not callable
. that stumped me for a while until i came across this :/ -
SeaWarrior404 about 5 yearsThis works! Make sure the spelling of Authorization is right. I used it as Authorisation and the request failed.
-
Wallem89 over 4 yearsYour anserd helped me but only after readin the link you provide which you came accros. Working with the HTTPBasicAuth import from requests.auth makes it very easy!