Python speech recognition for Raspberry Pi 2
Solution 1
I created a library called SpeakPython that helps Python developers do exactly this, and just released it under GPL3. The library is built upon pocketsphinx (sphinxbase) and gstreamer (for streaming recognition, which leads to fast results). It will allow you to attach python code to speech commands.
It's very accurate and dynamic for command parsing such as this, and I've tested it on the Pi already. Let me know if you have any issues.
Solution 2
Here is what I have up and running on my pi, it uses python speech recognition, pyaudio and pythons espeak for voice response (if you want that, if not just take it out) this will listen for voice input, print it to text and speak it back to you.. You can manipulate this to do whatever you want basically -
import pyaudio
from subprocess import call
import speech_recognition
r = sr.Recognizer()
r.energy_threshold=4000
with sr.Microphone(device_index = 2, sample_rate = 44100, chunk_size = 512) as source:
print 'listening..'
audio = r.listen(source)
print 'processing'
try:
message = (r.recognize_google(audio, language = 'en-us', show_all=False))
call(["espeak", message])
except:
call(['espeak', 'Could not understand you'])
Solution 3
To recognize few words on Raspberry Pi 2 with Python you can use Python bindings to Pocketsphinx
You can find pocketsphinx tutorial to get started here.
You can find some installation details for RPi here.
You can find code example here.
You can find already functioning example using pocketsphinx and python here.
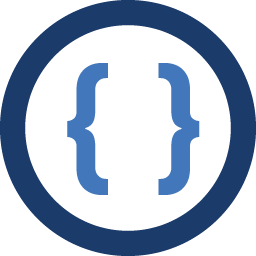
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to find a Speech recognition library similar to PySpeech that will work on a Raspberry Pi 2. I am new to this and have tried researching but there are so many applications I just need help choosing the correct one.
All I am trying to do is, when a user says something the program will recognize keywords and open up the correct part of my code which will just display information about that keyword.
Right now I am using Python 2.7 and PyQt4 to display what I want but am willing to change if there is something easier such as KivyPi, PyGame, etc. I am up for any ideas or any help to push me into the right direction.Thank You!