python zip & compress multiple files
10,586
Here is an example how to use the zipfile package with compression. Your code looks nearly okay. You have a typo in your list! And you shouldn't use try & except without specifying an error that you want to catch! I except the FileNotFoundError
that would occure if the file that you want to add to the zip doesn't exists.
Example (updated):
import zlib
import zipfile
def compress(file_names):
print("File Paths:")
print(file_names)
path = "C:/data/"
# Select the compression mode ZIP_DEFLATED for compression
# or zipfile.ZIP_STORED to just store the file
compression = zipfile.ZIP_DEFLATED
# create the zip file first parameter path/name, second mode
zf = zipfile.ZipFile("RAWs.zip", mode="w")
try:
for file_name in file_names:
# Add file to the zip file
# first parameter file to zip, second filename in zip
zf.write(path + file_name, file_name, compress_type=compression)
except FileNotFoundError:
print("An error occurred")
finally:
# Don't forget to close the file!
zf.close()
file_names= ["test_file.txt", "test_file2.txt"]
compress(file_names)
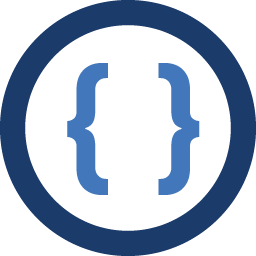
Author by
Admin
Updated on June 30, 2022Comments
-
Admin almost 2 years
I'm really sorry to be that newbie but I just can't figure out why this doesn't work by myself. There are already similar questions but they doesn't help at all. I try to zip & compress different files in a dir.
Here's my code:
import zipfile import zlib value_map = ['/home/shiva/Desktop/test2', 'None', False, False, True, False ['_MG_5290.JPG', '_MG_5294.JPG', '_MG_5293.JPG', '_MG_5295.JPG', '_MG_5291.JPG', 'IMG_5434.JPG', '_MG_5292.JPG'], ['_MG_5298.CR2', '_MG_5290.CR2', '_MG_5297.CR2', '_MG_5294.CR2', '_MG_5296.CR2', '_MG_5291.CR2', '_MG_5292.CR2', '_MG_5299.CR2', '_MG_5293.CR2', '_MG_5295.CR2']] def compress(value_map): print "value_map:" print value_map try: compression = zipfile.ZIP_DEFLATED zf = zipfile.ZipFile(value_map[0] + "/RAWs.zip", mode="w") for x in value_map[7]: print "test1" # prints zf.write(value_map[0] + x, compress_type=compression) # nope print "test2" # doesn't print zf.close() print ("[*] " + len(value_map[7]) + " have been moved to:") print ("[*] " + value_map[0] + "/RAWs.zip") except: print "[-] Couldn't compress\n[-] Exiting" sys.exit(0)
Just why???