Python3 tkinter set image size
28,982
Solution 1
As mentioned by several others, you should use PIL to resize your image before attaching it to a tkinter label:
from tkinter import Tk, Label
from PIL import Image, ImageTk
root = Tk()
img = ImageTk.PhotoImage(Image.open('img-path.png').resize(pixels_x, pixels_y)) # the one-liner I used in my app
label = Label(root, image=img, ...)
label.image = img # this feels redundant but the image didn't show up without it in my app
label.pack()
root.mainloop()
Solution 2
New syntax of resize:
resize((pixels_x, pixels_y))
So the code can be comething like this:
from tkinter import Tk, Label
from PIL import Image, ImageTk
root = Tk()
file = '/home/master/Work/Tensorflow/Project/Data/images/p001.png'
image = Image.open(file)
zoom = 1.8
#multiple image size by zoom
pixels_x, pixels_y = tuple([int(zoom * x) for x in image.size])
img = ImageTk.PhotoImage(image.resize((pixels_x, pixels_y)))
label = Label(root, image=img)
label.image = img
label.pack()
root.mainloop()
based on Nelson answer
Solution 3
You can add this one line if a simple zoom is acceptable :
image = PhotoImage(data=b64_data)
image = image.subsample(4, 4) # divide by 4
# image = image.zoom(2, 2) # zoom x 2
label = Label(image=image, bg="White")
Otherwise you should use the PIL lib which provide more accurate tools.
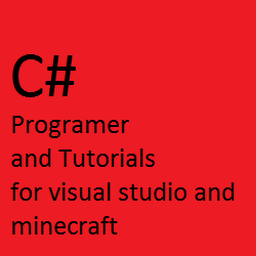
Author by
SeanOMik
Updated on April 27, 2021Comments
-
SeanOMik about 3 years
I have looked everywhere to find a way to set the size of an image. The image is set to a url. I have found other questions on the site but none of them have worked.
import urllib.request, base64 u = urllib.request.urlopen(currentWeatherIconURL) raw_data = u.read() u.close() b64_data = base64.encodestring(raw_data) image = PhotoImage(data=b64_data) label = Label(image=image, bg="White") label.pack()
That is the code that creates the image, how would I set the size of the image