Pyusb on windows - no backend available
Solution 1
Download and install libusb-win32-devel-filter-1.2.6.0.exe. It should work.
Solution 2
I had a similar issue recently trying to talk to a USB device I am developing. I scoured the web looking for libusb-1.0.dll's and had no luck. I found source code, but nothing built and ready to install. I ended up installing the libusb-win32 binaries, which is the libusb0.dll.
PyUSB will search for libusb-1.0, libusb0, and openUSB backends.
libusb0.dll was already on my system, but something was still not set up right, do PyUSB was not working.
I followed the directions here to download and install the driver using the GUI tools provided to install the filter driver, and the INF wizard. Note, it didn't work until I ran the INF wizard.
I'm pretty new to programming and I've found the lack of clear documentation/examples to string this all together rather frustrating.
Solution 3
I am using Python 2.6.5, libusb-win32-device.bin-0.1.12.1
and pyusb-1.0.0-a0
on a windows XP system and kept receiving ValueError: No backend available
.
Since there wasn't any real help on the web for this problem I spent a lot of time finding that ctypes util.py
uses the Path
variable to find the library file. My path
did not include windows\system32
and PYUSB
didn't find the library. I updated the path
variable and now the USB is working.
Solution 4
There's a simpler solution.
Download and unpack to C:\PATH the libusb-1.0.20 from download link
Then try this line:
backend = usb.backend.libusb1.get_backend(find_library=lambda x: "C:\PATH\libusb-1.0.20\MS32\dll\libusb-1.0.dll")
dev = usb.core.find(backend=backend, find_all=True)
Depending on your system, try either MS64 or MS32 version of the .dll
Update of 17/01/2020, after a request to share more code:
import usb.core
import usb.util
from infi.devicemanager import DeviceManager
dm = DeviceManager()
devices = dm.all_devices
for i in devices:
try:
print ('{} : address: {}, bus: {}, location: {}'.format(i.friendly_name, i.address, i.bus_number, i.location))
except Exception:
pass
import usb.backend.libusb1
backend = usb.backend.libusb1.get_backend(find_library=lambda x: "C:\\libusb-1.0.20\\MS32\\dll\\libusb-1.0.dll")
dev = usb.core.find(backend=backend, find_all=True)
def EnumerateUSB(): #I use a simple function that scans all known USB connections and saves their info in the file
with open("EnumerateUSBLog.txt", "w") as wf:
counter = 0
for d in dev:
try:
wf.write("USB Device number " + str(counter) + ":" + "\n")
wf.write(d._get_full_descriptor_str() + "\n")
wf.write(d.get_active_configuration() + "\n")
wf.write("\n")
counter += 1
except NotImplementedError:
wf.write("Device number " + str(counter) + "is busy." + "\n")
wf.write("\n")
counter += 1
except usb.core.USBError:
wf.write("Device number " + str(counter) + " is either disconnected or not found." + "\n")
wf.write("\n")
counter += 1
wf.close()
Solution 5
2021 and the problem still occurs on Windows (Windows 10). I solved it by installing pyusb
and libusb
and adding libusb path to Windows environment:
pip install pyusb
pip install libusb
-
libusb-1.0.dll
will be automatically added to:
\venv\Lib\site-packages\libusb\_platform\_windows\x64
and
\venv\Lib\site-packages\libusb\_platform\_windows\x32
- Now just add those paths (the full path) to Windows Path and restart CMD / PyCharm.
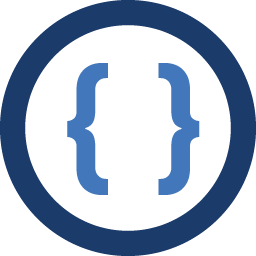
Admin
Updated on January 15, 2022Comments
-
Admin over 2 years
I'm trying to have my Python application interface with an NFC device via USB. The best option seems to be PyUSB, but I can't get it to connect to the libusb backend. I keep getting
ValueError: No backend available
I've looked at the stack trace, and found that
usb/backend/libusb10.py
(which is part of pyusb) is trying to loadlibusb-1.0.dll
to use as the backend, but it can't find it. It's not that it's not in my path, it's not on my computer at all!I have installed libusb-win32, but the resulting directory only seems to include libusb0.dll. Where is libusb-1.0.dll???!
I would love to know either where to get that dll, or even a different suggestion to get PyUSB to work on Windows 7.
-
JoSa about 11 yearsGiven that this is about XP (and the OP was asking Re: Windows 7) this might have been better as a comment.
-
Liviu over 9 yearsI added the current folder to the
PATH
. -
jonincanada over 8 yearsYes, run the inf-wizard and select your device to start.
-
Socre over 7 years@jonincanada it seems that i have run into the same problem as Garrett Hyde. but the difference is that i have downloaded and unzipped the file(libusb1.0.20),then copied a dll file, specifically(libusb-1.0.dll) and copied it to system 32; then i copied libusb-1.lib to python34/Lib. and still there is 'no backend' error,so can you help me solve the problem?
-
jjz about 4 yearsAdafruit has a good guide on getting pyusb working on Windows: learn.adafruit.com/circuitpython-on-any-computer-with-ft232h/…
-
Ashwin Kumar almost 3 yearsHave installed these packages and included the paths in Path Env Variable on my Win-10 machine. However, running this line of code still throws the "No Backenderror" import usb for dev in usb.core.find(find_all=True): print("Devices - " + dev)
-
Alaa M. almost 3 years@AshwinKumar - Are you sure you installed the correct libraries? There's a
pylibusb
which is not what you should install. Also, make sure to restart PyCharm / CMD after updating Windows Path (it won't work otherwise). Maybe try to start from a clean venv -
Ashwin Kumar almost 3 yearsI have installed the package you are mentioning. Here is the screenshot showing same - file.io/EYaucUUluN9e Have uploade the screenshot of my code here file.io/MyORGzN3zJF0 Please let me know if I am missing anything. You have to excuse any basic mistake - I am not an active developer :-)
-
Alaa M. almost 3 years@AshwinKumar - I can't open the links, either uploda to Imgur, or try to debug it by setting a breakpoint in
libloader.py
(located in/venv/Lib/site-packages/usb
) on line 92for candidate in candidates
, continue to the 2nd iteration untilcandidate == libusb-1.0
, step in tofind_library()
, and continue untildirectory
is the path you added to the Path, then see whatos.path.isfile(fname)
returns