quadratic formula with scanner inputs
Solution 1
I'm a little late to answer, but I corrected your problems (described in the other answers), fixed one of your calculations, and cleaned up your code.
import java.util.*;
public class Test {
public static void main(String[] args)
{
Scanner s = new Scanner(System.in);
System.out.println("Insert value for a: ");
double a = Double.parseDouble(s.nextLine());
System.out.println("Insert value for b: ");
double b = Double.parseDouble(s.nextLine());
System.out.println("Insert value for c: ");
double c = Double.parseDouble(s.nextLine());
s.close();
double answer1 = (-b + Math.sqrt(Math.pow(b, 2) - (4 * a * c))) / (2 * a);
double answer2 = (-b - Math.sqrt(Math.pow(b, 2) - (4 * a * c))) / (2 * a);
if (Double.isNaN(answer1) || Double.isNaN(answer2))
{
System.out.println("Answer contains imaginary numbers");
} else System.out.println("The values are: " + answer1 + ", " + answer2);
}
}
Solution 2
NaN
is something you get when the calculation is invalid. Such as dividing by 0 or taking the squareroot of -1.
When I test your code with a = 1
, b = 0
and c = -4
the answers is 2.02.0
The formatting is not right and the calculation of final2
is not negated.
Otherwise the code is right.
To improve you could check whether the discriminant is negative.
double d = b*b -4 * a * c;
if (d < 0){
System.out.println("Discriminant < 0, no real solutions" );
return;
}
double x1 = (-b -sqrt(d))/(2*a);
double x2 = (-b +sqrt(d))/(2*a);
System.out.format("The roots of your quadratic formula are %5.3f and %5.3f\n",x1,x2);
Or, if you prefer support for solutions from the complex domain:
if (d < 0) {
System.out.println("Discriminant < 0, only imaginary solutions");
double r = -b / (2 * a);
double i1 = -sqrt(-d) / (2 / a);
double i2 = sqrt(-d) / (2 / a);
System.out.format("The roots of your quadratic formula are (%5.3f + %5.3fi) and (%5.3f + %5.3fi)\n",r, i1, r, i2);
return;
}
Solution 3
You are getting NaN
because you are attempting to take the square root of a negative number. In math that's not allowed unless you are allowing complex numbers, e.g. 1 +/- 2i
.
This can happen in quadratic formulas when the discriminant (the thing in the square root) is negative, e.g. x^2 + 6*x + 100
: b^2 - 4ac
= 36 - 400 = -364. Taking the square root of a negative number in Java leads to NaN
. (not a number)
To test for NaN
, use Double.isNaN
and handle the NaN
appropriately.
In addition, your calculations are incorrect even if NaN
isn't being encountered:
$ java QuadraticFormulaSCN
insert value for a:
1
insert value for b:
5
insert value for C:
6
The x values are:-2.0-2.0
This should have outputted 2.0 and 3.0
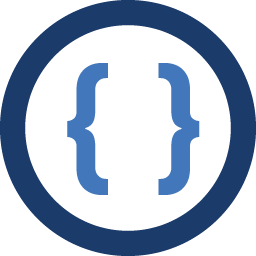
Admin
Updated on July 10, 2022Comments
-
Admin almost 2 years
Okay so I am a complete Java noob, and I'm trying to create a program for class that runs a quadratic equation using scanner inputs. So far what I've got is this:
import java.util.*; public class QuadraticFormulaSCN { public static void main(String[]args) { System.out.println("insert value for a:"); Scanner scan1 = new Scanner(System.in); double a = scan1.nextDouble(); System.out.println("insert value for b:"); Scanner scan2 = new Scanner(System.in); double b = scan2.nextDouble(); System.out.println("insert value for C:"); Scanner scan3 = new Scanner(System.in); double c = scan3.nextDouble(); double answer =((Math.sqrt(Math.pow(b,2)-(4*a*c))-b)/2); double final2 =(-b + Math.sqrt(Math.pow(b,2)-(4*a*c)))/2; System.out.println("The x values are:" + answer + final2); } }
But I get a weird output, specifically
NaNaN
... What do I do to fix this? What am I doing wrong?-
syb0rg about 11 yearsYou should only be using one
Scanner
. -
SJuan76 about 11 yearsAlso, it will help you if you (at least for testing purposes) avoid one-line code and separate the calculus in several variables, for ease of debug printing. Anyway, are you sure that the equation that you have set has at least one solution (and, IMHO, you should try to print both solutions if they do exist).
-