"connection.Children(0)" triggers "The enumerator of the collection cannot find en element with the specified index."
Solution 1
I am using the exact same script, which I found somewhere on the SAP help forums.
When I've encountered this issue before it's usually because the SAP GUI window was loaded AFTER lines
session.findById("wnd[0]/usr/txtRSYST-BNAME").text = "username"
session.findById("wnd[0]/usr/pwdRSYST-BCODE").text = "password"
There are two ways to fix this particular script. One is to add a MsgBox
that will pause the script, but give SAP GUI enough time to load. The other is to add WScript.Sleep(<a few seconds>)
to allow SAP GUI to load. Like so ...
Note that the below code has BOTH examples, but only 1 is necessary. I prefer the .Sleep()
because it requires no external input from a user.
If IsObject(WSHShell) Then
' Removed for clarity
End If
MsgBox "Click OK to continue" ' <-- MsgBox to pause script
WScript.Sleep(5000) ' <--- Wait 5 seconds for SAP GUI to load
If Not IsObject(application) Then
Set SapGuiAuto = GetObject("SAPGUI")
Set application = SapGuiAuto.GetScriptingEngine
End If
If Not IsObject(connection) Then
Set connection = application.Children(0)
End If
If Not IsObject(session) Then
Set session = connection.Children(0)
End If
If IsObject(WScript) Then
WScript.ConnectObject session, "on"
WScript.ConnectObject application, "on"
End If
session.findById("wnd[0]").resizeWorkingPane 164,40,false
session.findById("wnd[0]/usr/txtRSYST-BNAME").text = "username"
session.findById("wnd[0]/usr/pwdRSYST-BCODE").text = "password"
session.findById("wnd[0]/usr/pwdRSYST-BCODE").setFocus
session.findById("wnd[0]/usr/pwdRSYST-BCODE").caretPosition = 14
session.findById("wnd[0]").sendVKey 0
And of course, storing username and password in plain text is not a good practice. However, obfuscating passwords with the VBScript InputBox()
is not possible. You will have to use the command line, or create an IE object which is outside the scope of this question
Solution 2
Here's some VBS code that tries to wait for SAP to login and load properly. It worked well for me so far.
Function SAP_start_and_login(connection_string, use_sso, user, pass)
WScript.Echo "executing function SAP_start_and_login()"
REM Variables! Must declare before using because of Option Explicit
Dim WSHShell, SAPGUIPath
REM Main
Set WSHShell = WScript.CreateObject("WScript.Shell")
If IsObject(WSHShell) Then
REM Set the path to the SAP GUI directory
SAPGUIPath = "C:\Program Files (x86)\SAP\FrontEnd\SAPgui\"
REM Starts the SAP GUI
if use_sso then
WSHShell.Exec SAPGUIPath & "sapshcut.exe -client={your_client} -sysname=whatevah -gui=" & connection_string & " -snc_name={your_snc_name} -snc_qop={your_snc_qop}"
else
WSHShell.Exec SAPGUIPath & "sapshcut.exe -client={your_client} -sysname=whatevah -gui=" & connection_string & " -user=" & user & " -pw=" & pass
end if
REM WSHShell.Exec SAPGUIPath & "saplogon.exe """ & system & """"
Set WSHShell = Nothing
WScript.Echo "{waiting for SAP to finish loading..}"
SAP_start_and_login = WaitForSAP(connection_string, 50)
WScript.Sleep 1000
End If
End Function
Function CheckSapIsRunning(connection_string)
Running = False
Ready = False
If Not IsObject(application) Then
On Error Resume Next
Set SapGuiAuto = GetObject("SAPGUI")
If (Err.Number <> 0) Then
WScript.Echo "SAPGUI object not found yet"
' Error raised, object not found.
' Restore normal error handling.
On Error GoTo 0
Else
'WScript.Echo "einai ok"
' Object found.
' Restore normal error handling.
On Error GoTo 0
Set application = SapGuiAuto.GetScriptingEngine
If application.Connections.Count() > 0 Then
For i = 0 To (application.Connections.Count()-1)
REM WScript.Echo i
Set Connection = application.Children(0+i)
Conn = Connection.ConnectionString()
REM WScript.Echo Conn
If InStr(Conn, connection_string) Then
Running = True
Exit For
End If
Next
End If
If Running Then
REM WScript.Echo connection_string + " is running!"
REM WScript.Echo "sessions="+CStr(connection.Children.Count)
If Not IsObject(session) Then
if connection.Children.Count > 0 then
Set session = connection.Children(0)
If IsObject(WScript) Then
WScript.ConnectObject session, "on"
WScript.ConnectObject application, "on"
End If
If not session.Busy then
Ready=True
REM WScript.Echo connection_string + " session is ready!"
Dim sapWindow
set sapWindow = session.findById("wnd[0]", False)
if sapWindow is Nothing then
WScript.Echo connection_string + " sap window element not there yet"
else
WScript.Echo connection_string + " is loaded and visible!"
end if
Else
WScript.Echo connection_string + " session is busy!"
end if
else
WScript.Echo connection_string + " session not loaded yet!"
end if
End If
else
WScript.Echo connection_string + " not running!"
End If
End If
End If
REM WScript.Echo application.Connections.Count()
CheckSapIsRunning = Ready
End Function
Function WaitForSAP(connection_string, timeout)
counter = 0
returnValue = False
While NOT CheckSapIsRunning(connection_string) AND counter < timeout
counter = counter + 1
WScript.Sleep 1000
REM WScript.Echo connection_string + " is not ready"
WEnd
if counter = timeout then
WScript.Echo "timeout of " + CStr(timeout) + " seconds reached."
else
returnValue = True
end if
WaitForSAP = returnValue
End Function
This can be used like this
If SAP_start_and_login(connection_string, use_sso, username, password) Then
If Not IsObject(application) Then
Set SapGuiAuto = GetObject("SAPGUI")
Set application = SapGuiAuto.GetScriptingEngine
End If
...{the rest of your recorded script}
Else
WScript.Echo "Could not get a functioning SAP session"
WScript.Quit 1
End If
I'm using sapshcut.exe because it was easy to have both SSO and non SSO in a similar manner. The connection string is important in order to distinguish and identify the instance of SAP that you want to use in the rest of the script. It can be found from your current shortcut along with the SSO parameters that you might be using. If the SSO is true the user and pass parameters are not used. The timeout is also useful to avoid an endless loop if SAP is down or unreachable. This solution does not need a static sleep in the script in order to wait for SAP to load which could prevent issues if SAP took more than expected to load. Also no time is wasted if it is loaded sooner than expected.
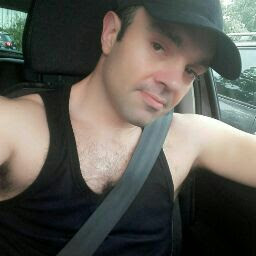
Comments
-
Malbordio almost 2 years
I am using a VBScript to login automatically into SAP GUI. It opens automatically the SAP GUI window, it loads the SAP server, but it doesn't populate automatically the user and password fields (remain blank).
It also gives a script error on line 52, char 4:
The enumerator of the collection cannot find en element with the specified index.
The code is the following:
REM The following script was written to log into the SAP server automatically. REM To view historical information and credit for this script please see REM the following thread on the SAP Community Network: REM http://scn.sap.com/thread/3763970 REM This script was last updated by Paul Street on 7/1/15 REM Directives Option Explicit REM Variables! Must declare before using because of Option Explicit Dim WSHShell, SAPGUIPath, SID, InstanceNo, WinTitle, SapGuiAuto, application, connection, session REM Main Set WSHShell = WScript.CreateObject("WScript.Shell") If IsObject(WSHShell) Then REM Set the path to the SAP GUI directory SAPGUIPath = "C:\Program Files (x86)\SAP\FrontEnd\SAPgui\" REM Set the SAP system ID SID = "eaiserver.domain.com" REM Set the instance number of the SAP system InstanceNo = "38" REM Starts the SAP GUI WSHShell.Exec SAPGUIPath & "SAPgui.exe " & SID & " " & _ InstanceNo REM Set the title of the SAP GUI window here WinTitle = "SAP" While Not WSHShell.AppActivate(WinTitle) WScript.Sleep 250 Wend Set WSHShell = Nothing End If REM Remove this if you need to test the above script and want a message box at the end launching the login screen. REM MsgBox "Here now your script..." If Not IsObject(application) Then Set SapGuiAuto = GetObject("SAPGUI") Set application = SapGuiAuto.GetScriptingEngine End If If Not IsObject(connection) Then Set connection = application.Children(0) End If If Not IsObject(session) Then Set session = connection.Children(0) End If If IsObject(WScript) Then WScript.ConnectObject session, "on" WScript.ConnectObject application, "on" End If session.findById("wnd[0]").maximize session.findById("wnd[0]/usr/txtRSYST-MANDT").Text = "100" session.findById("wnd[0]/usr/txtRSYST-BNAME").text = "I'veInsertedtheCorrectUsernameHere" session.findById("wnd[0]/usr/pwdRSYST-BCODE").text = "I'veInsertedtheCorrectPassHere" session.findById("wnd[0]/usr/txtRSYST-LANGU").Text = "PT" session.findById("wnd[0]/usr/pwdRSYST-BCODE").setFocus session.findById("wnd[0]/usr/pwdRSYST-BCODE").caretPosition = 10 session.findById("wnd[0]").sendVKey 0
Thanks for the help!
-
JackyBoi over 4 yearsCan you pls explain in a bit more detail on how to get started with this? I have a sapshortcut already on my desktop. So, how does this VBS script kick in?