"echo 1 > /sys/block/sdX/device/delete" on all disks except predetermined list
Here's an approach that should work:
Get the list of
sdX
devices to excludeexclude=$(cut -d/ -f3 exclude.txt)
Iterate over the
/sys/block/sdX
directories:for sysfile in /sys/block/sd? ; do
Extract the
sdX
name from that path, and build thedelete
file namedev=$(basename $sysfile) del=$sysfile/device/delete
Check if that
sdX
is in the excluded list:if [[ $exclude == *$dev* ]] ; then echo "Device $dev excluded"
Check if you have appropriate write permissions on the delete file
elif [ ! -w $del ] ; then echo "$del does not exist or is not writable"
Do the delete (not really)
else echo "echo 1 > $del" fi
You're done!
done
Related videos on Youtube
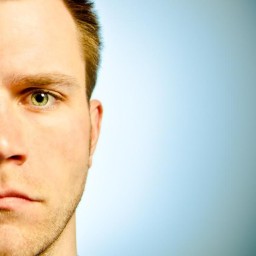
LVLAaron
Updated on September 18, 2022Comments
-
LVLAaron 8 months
I have a list of scsi disks that I need to remove. The list is considered random at best and changes from time to time. I want to remove everything except a predefined list that I have created. Let's assume for now that I only want to keep:
/dev/sda /dev/sdb
The command I need to execute is:
"echo 1 > /sys/block/sdX/device/delete"
Where X is the device to be removed.
I'm not good at bash scripting so I don't really know where to start.
To recap so I don't get DV'd for not being clear.
I need to "echo 1 > /sys/block/sdX/device/delete" for every sdX device on the system except for a predetermined list.
EDIT: After the answer below, this is what I've decided to use. "LocalDisks.txt" should contain lines like "/dev/sda"
#!/bin/bash exclude=$(cut -d/ -f3 LocalDisks.txt) for sysfile in /sys/block/sd* ; do dev=$(basename $sysfile) del=$sysfile/device/delete if [[ $exclude == *$dev* ]] ; then echo "Device $dev excluded" elif [ ! -w $del ] ; then echo "$del does not exist or is not writable" else echo 1 > $del fi done
-
LVLAaron over 11 yearsI do think that this will work out. However, I need to make one adjustment. I have so many disks that some are /dev/sdXX - if I add a question mark to the one you already have it only returns the /dev/sdXX and if there's only one question mark, it only returns the ones like /dev/sdX. Makes sense... How can it service both?
-
LVLAaron over 11 yearsI replaced the ? with a *
-
Mat over 11 yearsYep, that was the right thing to do there. I hadn't thought about what happens if you have a lot of drives.
-
LVLAaron over 11 yearsTechnically you gave me exactly what I asked for, I only specified one X. :)