"Iterating" through methods
Solution 1
There is a way using reflection:
try{
Method m= object.getClass().getMethod("getField"+String.valueOf(i), new Class[]{});
fields+=(String)m.invoke(object);
}catch(...){...}
However:
This business all smells of bad coding practice!
Can't you rewrite all the getFieldN()
methods like this?
String getField(int fieldNum)
You are asking for trouble by creating numbered methods. Remember that reflection is slow and should only be used when String-based method calls are absolutely essential to the flow of your program. I sometimes use this technique for user-defined scripting languages where you have to get a method by name. That isn't the case at all here, your calls are integer-indexed. You should therefore keep the integer as a parameter.
If this is legacy code and you are absolutely unable to change this bad coding, then you might be better off creating a new method getMethod(int)
as above to wrap the existing methods, that just delegates to the numbered getMethodN()
methods.
Solution 2
Class yourClass = YourClass.class;
for (Method method : yourClass.getMethods()){
method.invoke(obj, args);
}
See this guide for reference.
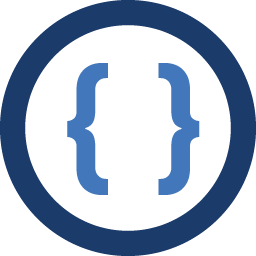
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
Let's say I've got a Java object that's got among others the following methods:
public String getField1(); public String getField2(); public String getField3(); public String getField4(); public String getField5();
Is there a way to iterate through these methods and call 'em like the following code?
String fields = ""; for(int i = 1; i <= 5; i ++){ fields += object.(getField+i) + " | "; }
Thank you for your upcoming ideas.
-
Admin almost 14 yearsI guess I forgot to mention, that my class has other methods too that I don't want to invoke. Would your code still do the job then?
-
Alberto Zaccagni almost 14 yearsIf you know their signatures you could call only them basing on
method.getName()
and onmethod.getParameterTypes()