"NoSuchWindowException: no such window: window was already closed" while switching tabs using Selenium and WebDriver through Python3
Solution 1
Thanks Debanjan (and apologies for late response - just got back to office). I was able to fix this by using
While True:
try:
[navigate to the new frame, wait for a specific element to show up]
break
except (NoSuchWindowException, NoSuchElementException):
pass
I had not realized that when I clicked to open the form, another window opened very briefly in the background, and then closed. This increased the window_handles to 2, so doing anything relating to waiting for 2 window handles still kept throwing an error.
Solution 2
As per your question and your code trials when you intend to navigate to a new tab you need to:
- Induce WebDriverWait while switching to the New Tab.
- Again when you intend to
switch()
to the desired<iframe>
you need to induce WebDriverWait again. - While you
switch()
to the desired<iframe>
try to use either ID, NAME, XPATH or CSS-SELECTOR of the desired<iframe>
. Your own code with these simple modification will be as follows:
from selenium import webdriver from selenium.webdriver.support import expected_conditions as EC from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.common.by import By url = "your_url" driver = webdriver.Chrome(executable_path = r'S:\Engineering\Jake\MasterControl Completing Pipette CalPM Forms\chromedriver') driver.get(url) windows_before = driver.current_window_handle driver.find_element_by_id('portal.scheduling.prepopulate').click() WebDriverWait(driver, 5).until(EC.number_of_windows_to_be(2)) windows_after = driver.window_handles new_window = [x for x in windows_after if x != windows_before][0] # driver.switch_to_window(new_window) <!---deprecated> driver.switch_to.window(new_window) WebDriverWait(driver, 5).until(EC.frame_to_be_available_and_switch_to_it((By.ID,"myframe"))) # or By.NAME, By.XPATH, By.CSS_SELECTOR
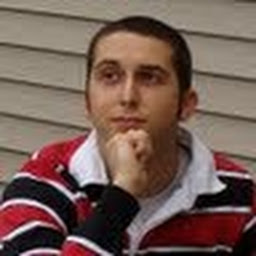
Jacob Miller
Updated on June 17, 2021Comments
-
Jacob Miller almost 3 years
I have a form that opens in a new tab when I click on it. When I try to navigate to that new tab, I keep getting a NoSuchWindowException. Code is pretty straightforward. 'myframe' is the frame within the new tab that the information will eventually get plugged into. Should I be waiting for something else?
from selenium import webdriver from selenium.webdriver.support import expected_conditions as EC from selenium.webdriver.support.ui import WebDriverWait, Select from selenium.webdriver.common.by import By from selenium.common.exceptions import TimeoutException, NoSuchElementException import time import pandas as pd url = ***** driver = webdriver.Chrome(executable_path = r'S:\Engineering\Jake\MasterControl Completing Pipette CalPM Forms\chromedriver') driver.get(url) wait = WebDriverWait(driver, 5) window_before = driver.window_handles[0] driver.find_element_by_id('portal.scheduling.prepopulate').click() window_after = driver.window_handles[1] driver.switch_to_window(window_after) driver.switch_to_default_content() wait.until(EC.frame_to_be_available_and_switch_to_it('myframe'))
Traceback (most recent call last): File "<ipython-input-308-2aa72eeedd51>", line 1, in <module> runfile('S:/Engineering/Jake/MasterControl Completing Pipette CalPM Forms/Pipette Completing CalPM Tasks.py', wdir='S:/Engineering/Jake/MasterControl Completing Pipette CalPM Forms') File "C:\ProgramData\Anaconda3\lib\site-packages\spyder_kernels\customize\spydercustomize.py", line 678, in runfile execfile(filename, namespace) File "C:\ProgramData\Anaconda3\lib\site-packages\spyder_kernels\customize\spydercustomize.py", line 106, in execfile exec(compile(f.read(), filename, 'exec'), namespace) File "S:/Engineering/Jake/MasterControl Completing Pipette CalPM Forms/Pipette Completing CalPM Tasks.py", line 150, in <module> create_new_cal_task(asset_number) File "S:/Engineering/Jake/MasterControl Completing Pipette CalPM Forms/Pipette Completing CalPM Tasks.py", line 130, in create_new_cal_task driver.switch_to_default_content() File "C:\ProgramData\Anaconda3\lib\site-packages\selenium\webdriver\remote\webdriver.py", line 783, in switch_to_default_content self._switch_to.default_content() File "C:\ProgramData\Anaconda3\lib\site-packages\selenium\webdriver\remote\switch_to.py", line 65, in default_content self._driver.execute(Command.SWITCH_TO_FRAME, {'id': None}) File "C:\ProgramData\Anaconda3\lib\site-packages\selenium\webdriver\remote\webdriver.py", line 314, in execute self.error_handler.check_response(response) File "C:\ProgramData\Anaconda3\lib\site-packages\selenium\webdriver\remote\errorhandler.py", line 242, in check_response raise exception_class(message, screen, stacktrace) NoSuchWindowException: no such window: window was already closed (Session info: chrome=68.0.3440.106) (Driver info: chromedriver=2.40.565498 (ea082db3280dd6843ebfb08a625e3eb905c4f5ab),platform=Windows NT 6.1.7601 SP1 x86_64)