"querySnapshot.forEach is not a function" in firestore
13,084
Solution 1
import { firestore } from "../../firebase";
export const loadCategories = () => {
return (dispatch, getState) => {
firestore
.collection("CATEGORIES")
.get()
.then((querySnapshot) => {
if (!querySnapshot.empty) {
querySnapshot.forEach((doc) => {
console.log(doc.id, "=>", doc.data());
});
}
})
.catch((error) => {
console.log(error);
});
};
};
Solution 2
I have a collection of users including uid
just like yours. And for each user, it contains a sub-collection called friends
.
Currently, I'm using the following code for my project without having any issues.
module.exports = ({ functions, firestore }) => {
return functions.firestore.document('/users/{uid}').onDelete((event) => {
const userFriendsRef = getFriendsRef(firestore, uid);
userFriendsRef.get().then(snapshot => {
if (snapshot.docs.length === 0) {
console.log(`User has no friend list.`);
return;
} else {
snapshot.forEach(doc => {
// call some func using doc.id
});
}
}
}
};
function getFriendsRef(firestore, uid) {
return firestore.doc(`users/${uid}`).collection('friends');
}
Give it a try to fix your code from
db.collection('users').doc(currentUser.uid).collection('roles')
to
db.doc(`users/${currentUser.uid}`).collection('roles')
Solution 3
It is not clear what you are doing with the rol
and status
variables. You have declared them as though you are storing a single value, yet you are returning an array of roles and iterating through them.
With regards to getting the results, if your browser supports ES6, then you could do the following:
let userRef1 = db.collection(`users/${currentUser.uid}/roles`)
let cont = 0
let rol;
let rolStatus;
return userRef1.get()
.then(querySnapshot => {
// Check if there is data
if(!querySnapshot.empty) {
// Create an array containing only the document data
querySnapshot = querySnapshot.map(documentSnapshot => documentSnapshot.data());
querySnapshot.forEach(doc => {
let {rol, status} = doc;
console.log(`rol: ${rol} - status: ${status}`);
});
} else {
console.log('No data to show');
}
})
.catch(err => {
console.error(err);
});
Please note: I've only tested this with the Node SDK
Solution 4
// Firebase App (the core Firebase SDK) is always required and must be listed first
import * as firebase from "firebase/app";
// Add the Firebase products that you want to use
import "firebase/auth";
import "firebase/firestore";
const firebaseConfig = {
apiKey: "AIzaSyDNdWutrJ3Axpm-8ngNhzkzcw1g3QvkeFM",
authDomain: "books-7bd8b.firebaseapp.com",
databaseURL: "https://books-7bd8b.firebaseio.com",
projectId: "books-7bd8b",
storageBucket: "books-7bd8b.appspot.com",
messagingSenderId: "385706228283",
appId: "1:385706228283:web:a3c2c9585dd74d54693a1e",
};
firebase.initializeApp(firebaseConfig);
export const firebaseAuth = firebase.auth();
export const firestore = firebase.firestore();
export default firebase;
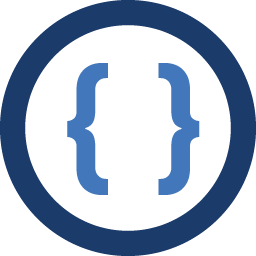
Author by
Admin
Updated on June 13, 2022Comments
-
Admin about 2 years
I'm trying to do a query to the database, to get all documents of sub-collection "roles" to redirect to different routes.
let userRef1 = db.collection('users').doc(currentUser.uid).collection('roles') let cont = 0 let rol = '' let rolStatus = '' userRef1.get().then(function(querySnapshot) { querySnapshot.forEach(function(doc) { cont++ rol = doc.data().rol rolStatus = doc.data().status });
-
Jason Berryman over 6 yearsYou can shorten this further to
let userRef1 = db.collection(<bt>users/${currentUser.uid}/roles<bt>)
. I can't format this properly in a comment. Please replace <bt> with a backtick -
Ivo Mori almost 4 yearsWelcome to Stack Overflow. Note that you can edit your answer; there's no need to post another answer for the same question. I suggest you either remove this answer or your other answer and then edit the remaining answer as needed. Also, it's much better to enhance your code-only-answer with some explanations. Have a look at How do I write a good answer?.