"slash before every quote" problem
Solution 1
Looks like you have magic quotes turned on. Use below condition using stripslashes
with whatever text you want to process:
if(get_magic_quotes_gpc())
{
$your_text = stripslashes($your_text);
}
Now you can process $your_text
variable normally.
Update:
Magic quotes are exaplained here. For well written code there is normally no harm in disabling it.
Solution 2
You likely have magic quotes turned on. You need to stripslashes()
it as well.
Nicest way would be to wrap this in a function:
function get_string($array, $index, $default = null) {
if (isset($array[$index]) && strlen($value = trim($array[$index])) > 0) {
return get_magic_quotes_gpc() ? stripslashes($value) : $value;
} else {
return $default;
}
}
Which you can use as
$annonsera_headline = get_string($_POST, 'annonsera_headline');
By the way:
And if I don't even use htmlentities then everything after the quotes dissappears.
It's actually still there in the HTML source, you only don't see it. Do a View Source ;)
Update as per your update: the magic quotes is there to prevent SQL injection attacks in code of beginners. You see this often in 3rd party hosts. If you really know what you're doing in the code, then you can safely turn it off. But if you want to make your code distributable, then you'll really take this into account when gathering request parameters. For this the above function example is perfectly suitable (you only need to write simliar get_boolean()
, get_number()
, get_array()
functions yourself).
Solution 3
Yes, you should disable magic quotes if you can. The feature is deprecated, and will likely go away completely in the future.
If you've relied on magic quotes for escaping data (for instance when inserting it into a database) you will may be opening yourself up to sql injection vulnerabilities if you disable it. You should check all your queries and make sure you're using mysql_real_escape_string()
.
I include the following file to undo magic quotes in apps that are deployed to servers not under my control.
<?php
set_magic_quotes_runtime(0);
function _remove_magic_quotes(&$input) {
if(is_array($input)) {
foreach(array_keys($input) as $key) _remove_magic_quotes($input[$key]);
}
else $input = stripslashes($input);
}
if(get_magic_quotes_gpc()) {
_remove_magic_quotes($_REQUEST);
_remove_magic_quotes($_GET);
_remove_magic_quotes($_POST);
_remove_magic_quotes($_COOKIE);
}
return true;
?>
Solution 4
This is actually a function of PHP trying to be security conscious, luckily there is an easy fix for it that looks something like this:
if (get_magic_quotes_gpc()) {$var = stripslashes($var);}
There isn't a huge problem in having it enabled, it comes down to personal preference. If you code will be moving servers much and you can't disable it through your php.ini file, it's best to use something as described above.
If you have access to your php.ini file and you want to change it, because you don't want to have to validate it each time you can add the following line to php.ini
magic_quotes_gpc = Off
Or the following to your .htaccess:
php_flag magic_quotes_gpc Off
Hope this helps clear things up.
Solution 5
Looks like your server is setup to use Magic Quotes.
You can fix it by stripping them with stripslashes
, or better, by turning off Magic Quotes.
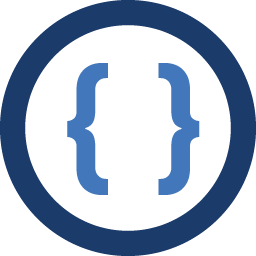
Admin
Updated on July 07, 2022Comments
-
Admin almost 2 years
I have a php page which contains a form.
Sometimes this page is submitted to itself (like when pics are uploaded).
I wouldn't want users to have to fill in every field again and again, so I use this as a value of a text-input inside the form:
value="<?php echo htmlentities(@$_POST['annonsera_headline'],ENT_COMPAT,'UTF-8');?>">
This works, except it adds a "\" sign before every double-quote...
For instance writing 19" wheels gives after page is submitted to itself:
19\" wheels
And if I don't even use htmlentities then everything after the quotes dissappears.
What is the problem here?
UPDATE:
Okay, so the prob is magic_quotes... This is enabled on my server...
Should I disable it? I have root access and it is my server :)
Whats the harm in disabling it?
-
Admin almost 14 yearswhat should I use instead of htmlentities then?
-
Morfildur almost 14 yearsyou should always use htmlentities if the content might come from userinput (as
$_POST['annonsera_headline']
obviously does), even if it passed through a database. If you don't then you get a cross-site scripting (XSS) vulnerability as people might find a way to inject javascript or their own HTML into the page, possibly even redirecting the form input (which is especially evil for logins). -
Your Common Sense almost 14 years@oezi what's wrong with htmlentities()?
-
oezi almost 14 yearsit's the VALUE of an INPUT FIELD. if you use htmlentities in this case, you will see things like & or ä there, wich is not what it should be! XSS isn't possible from an input value. thanks a lot for a senseless downvote...
-
Dominic Rodger almost 14 yearsPer my second sentence- turn off Magic Quotes! They're evil.
-
Rob almost 14 years@Camran for text field data I use
function dequote($instr) { return str_replace("'", ''', str_replace('"', '"', $instr)); }
-
Your Common Sense almost 14 years
$var='Bill "buffalo" Tuckson'; <input type="<?php echo $var?>">
. What will you see in the field? -
Your Common Sense almost 14 years@Rob don't be silly, use
htmlspecialchars()
-
Your Common Sense almost 14 yearsnot that evil. just litter your data about