"The final argument passed to useEffect changed size between renders", except I don't think it does?
Solution 1
If you have a
useEffect(() => {}, itemArray)
and you modify itemArray, you will get this error. this is probably just a typo. I did this when I had a list of items I was using from state. It didn't catch it because it was an array. You need [] around the itemArray.
useEffect(() => {}, [itemArray])
The real issue is that your dependency array changed size, not that you added another item to your array. fix this typo and you should be fine.
Solution 2
You can try this
useEffect(() => {
}, [itemList])
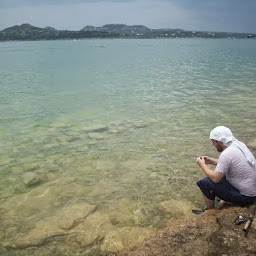
Chris Foster
Updated on December 03, 2021Comments
-
Chris Foster over 2 years
I don't think I've ever received this error before:
The final argument passed to useEffect changed size between renders. The order and size of this array must remain constant.
I've done axios requests in
useEffect()
100 times, usinguseEffect()
in a similar manner tocomponentDidMount()
, but this is the first time that I've used a reusable function withasync
/await
, and resolved the data back to theuseEffect()
. Other people online are doing this exact same thing in their tutorials, but they never mentioned this error.const [tableData, setTableData] = useState([]) useEffect(() => { const data = async() => { const dataArr = await getPagList('tags', 1, 25) console.log("Data received: ", dataArr) if(dataArr.length > 0) { setTableData(dataArr) } } data() }, [])
I believe, it's complaining about the empty array I'm feeding
useEffect()
as the 2nd parameter. However, that empty array isn't changing... Right? I tried googling it, but the solutions I found for this error were what I'm already doing. None of the examples were usingasync
/await
.I have also tried this, with no success:
useEffect(() => { setData() }, []) const setData = () => { const data = async() => { const dataArr = await getPagList('tags', 1, 25) console.log("Data received: ", dataArr) if(dataArr.length > 0) { setTableData(dataArr) } // TODO Properly handle this data now that I'm getting it in. It's only 9 records though. } data() }
Am I not exiting the useEffect properly or something? Anyway, thanks guys.
-
AshTyson almost 3 yearsEven if the dependency array is empty this warning is thrown. Can anyone shed some light on this?
-
Jason G almost 3 years@AshTyson what do you mean? useEffect(() => {}, []) does not throw an error.
-
AshTyson almost 3 yearsNo there are parts of our application it does throw this warning. Ofcourse we dont have a empty function as the first parameter
-
Jason G almost 3 yearsI'm not sure what you mean then. show some code and i can take a look
-
AshTyson almost 3 yearsCan't really post it all here because its proprietary. But here are some screenshots. React is very weird. ibb.co/xjJvv0x ibb.co/xJ4RpFQ it threw 21 errors for the same line.
-
Jason G almost 3 yearswell, archiveId needs to be in the list to prevent the linting error. I'm not sure without seeing the rest of the code what is going on.
-
AshTyson almost 3 yearsThat specific rule is disabled as you can see from the comment. This is the only useEffect in that file. And it throws this error multiple times. Thats why I say React doesn't handle/bubble errors very well.