"ViewPostImeInputStage processPointer 0" in log when item in RecyclerView is tapped?
10,542
You are not calling the activity in the RecyclerView on touch event.
The code with the correction is:
mRecyclerView.addOnItemTouchListener(new RecyclerView.OnItemTouchListener() {
@Override
public boolean onInterceptTouchEvent(RecyclerView recyclerView, MotionEvent motionEvent) {
View child = recyclerView.findChildViewUnder(motionEvent.getX(), motionEvent.getY());
if (child != null && mGestureDetector.onTouchEvent(motionEvent)) {
int position = recyclerView.getChildLayoutPosition(child);
Note selectedNote = mNotes.get(position);
Intent editorIntent = new Intent(getActivity(), NoteEditorActivity.class);
editorIntent.putExtra("id", selectedNote.getId());
startActivity(editorIntent);
}
System.out.print("Failed to load Editor class.");
return false;
}
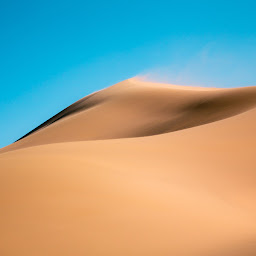
Author by
George B
Updated on June 15, 2022Comments
-
George B almost 2 years
In my app I am creating, I have a list of items. When a user taps on one of these items, a new window is supposed to open, displaying the information inside the item that the user tapped on. Here is my java code for that-
NoteListFragment.java
public class NoteListFragment extends Fragment { private FloatingActionButton mFab; private View mRootView; private List<Note> mNotes; private RecyclerView mRecyclerView; private NoteListAdapter mAdapter; private RecyclerView.LayoutManager mLayoutManager; public NoteListFragment() { // Required empty public constructor } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment and hold the reference //in mRootView mRootView = inflater.inflate(R.layout.fragment_note_list, container, false); //Get a programmatic reference to the Floating Action Button mFab = (FloatingActionButton)mRootView.findViewById(R.id.fab); //attach an onClick listener to the Floating Action Button mFab.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { startActivity(new Intent(getActivity(), NoteEditorActivity.class)); } }); setupList(); return mRootView; } private void setupList() { mRecyclerView = (RecyclerView) mRootView.findViewById(R.id.note_recycler_view); mRecyclerView.setHasFixedSize(true); mLayoutManager = new LinearLayoutManager(getActivity()); mRecyclerView.setLayoutManager(mLayoutManager); final GestureDetector mGestureDetector = new GestureDetector(getActivity(), new GestureDetector.SimpleOnGestureListener(){ @Override public boolean onSingleTapUp(MotionEvent e) { return true; } }); mRecyclerView.addOnItemTouchListener(new RecyclerView.OnItemTouchListener() { @Override public boolean onInterceptTouchEvent(RecyclerView recyclerView, MotionEvent motionEvent) { View child = recyclerView.findChildViewUnder(motionEvent.getX(), motionEvent.getY()); if (child != null && mGestureDetector.onTouchEvent(motionEvent)) { int position = recyclerView.getChildLayoutPosition(child); Note selectedNote = mNotes.get(position); Intent editorIntent = new Intent(getActivity(), NoteEditorActivity.class); editorIntent.putExtra("id", selectedNote.getId()); } System.out.print("Failed to load Editor class."); return false; } @Override public void onTouchEvent(RecyclerView rv, MotionEvent e) { } @Override public void onRequestDisallowInterceptTouchEvent(boolean disallowIntercept) { } }); mAdapter = new NoteListAdapter(mNotes, getActivity()); mRecyclerView.setAdapter(mAdapter); mNotes = NoteManager.newInstance(getActivity()).getAllNotes(); } }
NoteEditorActivity.java
public class NoteEditorActivity extends AppCompatActivity { private Toolbar mToolbar; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_note_editor); mToolbar = (Toolbar)findViewById(R.id.toolbar); setSupportActionBar(mToolbar); getSupportActionBar().setDisplayHomeAsUpEnabled(true); //remove this line in the MainActivity.java if (savedInstanceState == null){ Bundle args = getIntent().getExtras(); if (args != null && args.containsKey("id")){ long id = args.getLong("id", 0); if (id > 0){ openFragment(NotePlainEditorFragment.newInstance(id), "Editor"); } } openFragment(NotePlainEditorFragment.newInstance(0), "Editor"); } } @Override public boolean onCreateOptionsMenu(Menu menu) { // Inflate the menu; this adds items to the action bar if it is present. getMenuInflater().inflate(R.menu.menu_note_editor, menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { // Handle action bar item clicks here. The action bar will // automatically handle clicks on the Home/Up button, so long // as you specify a parent activity in AndroidManifest.xml. int id = item.getItemId(); //noinspection SimplifiableIfStatement if (id == R.id.action_settings) { return true; } return super.onOptionsItemSelected(item); } private void openFragment(final Fragment fragment, String title){ getSupportFragmentManager() .beginTransaction() .setTransition(FragmentTransaction.TRANSIT_FRAGMENT_OPEN) .replace(R.id.container, fragment) .addToBackStack(null) .commit(); getSupportActionBar().setTitle(title); } }
For some reason, when I tap one of the items, nothing happens, and the log says
11-26 09:45:17.963 12963-12963/com.example.simplenotepad D/ViewRootImpl: ViewPostImeInputStage processPointer 0 11-26 09:45:18.073 12963-12963/com.example.simplenotepad D/ViewRootImpl: ViewPostImeInputStage processPointer 1
What does this mean? How do I fix this? Thanks!
-
George B over 7 yearsNow the editor screen does come up, but it doesn't display any information. You did answer my question though.
-
jonathanrz over 7 years@GeorgeB if It is not showing, the problem is in the NoteEditorActivity. Can you post the code of NoteEditorActivity?
-
George B over 7 yearsI've posted it in the question now
-
jonathanrz over 7 years@GeorgeB the id of selected node is a long? Can you debug or add logs to see if the args from intent are ok?