R: append a "character" type to a list of numeric values
Solution 1
@Fernando pretty much has the idea right: your "row" should be a list
if you want to combine it with a data.frame
.
Here are a couple of examples:
## A sample `data.frame`
mydf <- data.frame(A = c("one", "two"), B = 1:2, C = 3:4,
D = 5:6, stringsAsFactors = FALSE)
## Your initial vector...
addMe <- c(1, 10, 12)
## ... now as a list
addMe <- c("name", as.list(addMe))
addMe
# [[1]]
# [1] "name"
#
# [[2]]
# [1] 1
#
# [[3]]
# [1] 10
#
# [[4]]
# [1] 12
#
## Put it all together...
new <- rbind(mydf, addMe)
new
# A B C D
# 1 one 1 3 5
# 2 two 2 4 6
# 3 name 1 10 12
str(new)
# 'data.frame': 3 obs. of 4 variables:
# $ A: chr "one" "two" "name"
# $ B: num 1 2 1
# $ C: num 3 4 10
# $ D: num 5 6 12
Alternatively, create a one row (or more, if necessary) data.frame
and bind that with the existing data.frame
:
addMe <- c(1, 10, 12)
rbind(mydf, setNames(cbind("name", data.frame(t(addMe))), names(mydf)))
# A B C D
# 1 one 1 3 5
# 2 two 2 4 6
# 3 name 1 10 12
Major note: This depends on your first column being a character, not factor, variable. If they are factors, you need to make sure the variable contains the right levels
(or convert to character first, then re-factor).
Solution 2
How about using the list element name for the string:
l <- list('name'=c(1,10,12,24))
l
## $name
## [1] 1 10 12 24
names(l)
## [1] "name"
Extract the numeric vector by name:
l[["name"]]
## [1] 1 10 12 24
Make this into a data frame:
as.data.frame(l)
## name
## 1 1
## 2 10
## 3 12
## 4 24
Solution 3
You' using a vector
, not a list
. You cannot mix types in vectors, use a list
instead:
my.list = list(1,10,12,24)
my.list = append("name", my.list)
And remember that 'growing' structures in R is very bad for performance.
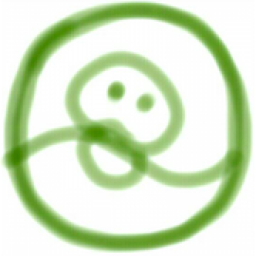
Comments
-
biohazard 6 months
I have a list of numeric values, say
> list <- c(1,10,12,24) > list [1] 1 10 12 24
and I want to append a string of characters to it so that the output reads:
> list [1] "name" 1 10 12 24
I tried the following code:
list <- c("name",1,10,12,24)
or
list <- c(1,10,12,24) list <- c("name",as.numeric(list))
but, predictably, all the numerics were converted to a character type:
> list <- c(1,10,12,24) > list <- c("name",as.numeric(list)) > list [1] "name" "1" "10" "12" "24"
How can I preserve the numeric type while adding a character type in front?
[EDIT] The goal is to add this as a row to an existing data frame df where df[1] contains strings and df[2:5] contain numerics.
-
Matthew Lundberg over 9 yearsYou want to create a vector that contains a string and numerics. That's not possible. How about using the list element name?
-
Roland over 9 yearsWhy do you want this? There's probably a much better alternative.
-
biohazard over 9 yearsI want to add this as a new row in a data frame, where the first column are names and the other columns contain numerics...
-
Matthew Lundberg over 9 years@biohazard Then you want to name the elements of the list, not add a name to the vector.
-
-
Matthew Lundberg over 9 yearsGiven the question's latest edit, I'm not sure that this really answers the question.
-
biohazard over 9 yearsThat's right, I wasn't able to append it as a row to my data frame (even by using
t(as.data.frame(l)
)... so I gave up and added the row by hand in the raw data and re-imported it ^^; but your answer still taught me something! -
A5C1D2H2I1M1N2O1R2T1 over 9 years+1. The problem pretty much boils down to this issue. It would have been nice to see a more fleshed out answer though.