Radio Buttons to select specific text inputs during checkout in WooCommerce
In woocommerce the is no hook to change your form in this way.
1. You will have to add the fields to form-billing.php (copy woocommerce/templates/chechout/form-billing.php to {yourtheme}/checkout/form-billing.php). This file contains a foreach-loop which add your fields to the form. Add your custom fields in or after this loop. Example:
<?php foreach ($checkout->checkout_fields['billing'] as $key => $field) : ?>
<?php woocommerce_form_field( $key, $field, $checkout->get_value( $key ) ); ?>
<?
if($key=='billing_first_name')
{
?>
<input type="radio" name="gender" value="female">Female
<input type="radio" name="gender" value="male">Male
<?
}
?>
<?php endforeach; ?>
You can also add jQuery here (after the foreach loop) to show / hide some fields based on the selecting of the user. Example:
<script type="text/javascript">
jQuery('input[name="gender"]').live('change', function(){ alert('gender checked');});
</script>
2 Check the input of your custom field(s) after submit. Add this to your functions.php example:
/**
* Process the checkout
**/
add_action('woocommerce_checkout_process', 'my_custom_checkout_field_process');
function my_custom_checkout_field_process() {
global $woocommerce;
if($_POST['gender']=='male')
{
$woocommerce->add_error( 'You are a female' );
}
}
3 add the input to the database, example:
/**
* Update the order meta with field value
**/
add_action('woocommerce_checkout_update_order_meta', 'my_custom_checkout_field_update_order_meta');
function my_custom_checkout_field_update_order_meta( $order_id ) {
if ($_POST['gender']) update_post_meta( $order_id, 'Gender', esc_attr($_POST['gender']));
}
See also: https://gist.github.com/mikejolley/1604009 for more example code
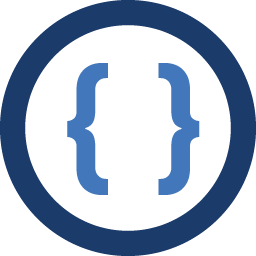
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I am adding custom fields to my checkout area in WooCommerce through my child theme's functions.php. I have added some text inputs, but I need to add two radio buttons that will select/ activate two seperate "sets" of text inputs.
Basically I need a way for my customers to be able to select Button A: enter payment info OR select Button B: and enter a PO Number. I need to make sure that the button that is NOT selected will not write to the database and hold up / produce errors during checkout.
If you go to the products page and add a product, then navigate to the checkout area you can see what I have already done. The code is below:
add_action('woocommerce_after_order_notes', 'custom_checkout_field'); function custom_checkout_field( $checkout ) { echo '<div id="payment_info"><h3>'.__('Payment Information').'</h3>'; woocommerce_form_field( 'credit_card_name', array( 'type' => 'text', 'class' => array('form-row-wide'), 'label' => __('Name on Credit Card'), 'required' => true, 'placeholder' => __(''), ), $checkout->get_value( 'credit_card_name' )); woocommerce_form_field( 'card_type', array( 'type' => 'select', 'class' => array(''), 'label' => __('Card Type'), 'required' => true, 'placeholder' => __(''), 'options' => array( 'American Express' => __('American Express'), 'Discover' => __('Discover'), 'Mastercard' => __('Mastercard'), 'Visa' => __('Visa') )), $checkout->get_value( 'card_type' )); woocommerce_form_field( 'card_number', array( 'type' => 'text', 'class' => array(''), 'label' => __('Card Number'), 'required' => true, 'placeholder' => __('XXXX-XXXX-XXXX-XXXX'), ), $checkout->get_value( 'card_number' )); woocommerce_form_field( 'card_expiration_month', array( 'type' => 'select', 'class' => array('form-row-first'), 'label' => __('Expiration Month'), 'required' => true, 'placeholder' => __('- Select Month -'), 'options' => array( '1' => __('1'), '2' => __('2'), '3' => __('3'), '4' => __('4'), '5' => __('5'), '6' => __('6'), '7' => __('7'), '8' => __('8'), '9' => __('9'), '10' => __('10'), '11' => __('11'), '12' => __('12') )), $checkout->get_value( 'card_expiration_month' )); woocommerce_form_field( 'card_expiration_year', array( 'type' => 'select', 'class' => array('form-row-last'), 'label' => __('Expiration Year'), 'required' => true, 'placeholder' => __('- Select Year -'), 'options' => array( '2013' => __('2013'), '2014' => __('2014'), '2015' => __('2015'), '2016' => __('2016'), '2017' => __('2017'), '2018' => __('2018'), '2019' => __('2019'), '2020' => __('2020') )), $checkout->get_value( 'card_expiration_year' )); woocommerce_form_field( 'security_code', array( 'type' => 'text', 'class' => array('form-row-first'), 'label' => __('Security Code'), 'required' => true, 'placeholder' => __(''), ), $checkout->get_value( 'security_code' )); woocommerce_form_field( 'order_number', array( 'type' => 'text', 'class' => array('form-row-wide'), 'label' => __('PO Number (if required)'), 'placeholder' => __(''), ), $checkout->get_value( 'order_number' )); echo '</div>';
So it needs to look like this:
Payment Information
[x] Credit Card
Name [ __ active input area __ ]
Card Number [ __ active input area __ ] etc.[ _ ] PO Number
Enter Number [ __ inactive input area __ ]