Rails - how do you display data from the database
Solution 1
Alternatively in your view:
<% User.all.each do |user| %>
<%= user.id %>
<% end %>
This will print out the user_id for all the records in User
Solution 2
If you only want a UID for a user object it's fairly standard to let rails sort this out for you, so you just generate the required attributes of your table and don't include user_id. However it might be the case you want this user_id in which case ignore what I just said. You don't need the has_one relation unless you have another model named user_id (which is possible I guess)
The index method is generally meant to return all users, or at least a subset (if you use pagination for example) I would therefore recommend using the following in an index method
User.find :all
Notice I have left the brackets off, it doesn't make a difference but is a rails convention (it make it looks more like a DSL).
To return 1 user you might want a method like this
# GET /posts/1
def show
@user = User.first
end
But convention states the id would be specified by url sent, so it might look more like this
# GET /posts/1
def show
@user = User.find params[:id]
end
if you then access a url like the one specified above the show method (/posts/1, where 1 is the ID)
The view for this method should look something like this:
<%= @user.user_id %>
Note the @user is a member variable passed to the view by the show method, in this case an instance of a user with the id 1, and you are accessing the user_id attribute of that instance so you don't need the User.
as this would be calling on a class method not a method of an instance of the user object.
To answer your specific question it should look more like
Model
class User < ActiveRecord::Base
set_primary_key :user_id
end
Controller
class HomeController < ApplicationController
def show
@user = User.first
end
end
Accessing the show view with /users/0
The View would contain
<%= @user.user_id %>
EDIT:
To display the user logged in you will probably need a user session object which is created when a user logs in and destroyed when the user logs out. The user session object should have an associated user object, you can then query the user_session object for which user is logged.
I strongly recommend using authlogic, it's simple enough to know whats going on but does the trick
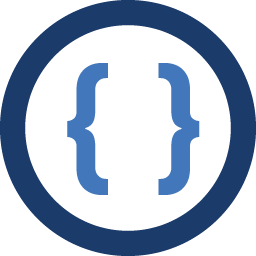
Admin
Updated on September 01, 2020Comments
-
Admin over 3 years
Im fairly new to Rails and i am simply trying to display the user_id of a record in a sqlite3 database on a page. Here is my code:
Model
class User < ActiveRecord::Base set_primary_key :user_id has_one :user_id end
Controller
class HomeController < ApplicationController def index @users = User.find(0) end end
View
<%= User.users.user_id %>
Im sure im making a silly mistake.