rails simple_form - hidden field - create?
Solution 1
try this
= f.input :title, :as => :hidden, :input_html => { :value => "some value" }
Solution 2
Shortest Yet !!!
=f.hidden_field :title, :value => "some value"
Shorter, DRYer and perhaps more obvious.
Of course with ruby 1.9 and the new hash format we can go 3 characters shorter with...
=f.hidden_field :title, value: "some value"
Solution 3
Correct way (if you are not trying to reset the value of the hidden_field input) is:
f.hidden_field :method, :value => value_of_the_hidden_field_as_it_comes_through_in_your_form
Where :method
is the method that when called on the object results in the value you want
So following the example above:
= simple_form_for @movie do |f|
= f.hidden :title, "some value"
= f.button :submit
The code used in the example will reset the value (:title) of @movie being passed by the form. If you need to access the value (:title) of a movie, instead of resetting it, do this:
= simple_form_for @movie do |f|
= f.hidden :title, :value => params[:movie][:title]
= f.button :submit
Again only use my answer is you do not want to reset the value submitted by the user.
I hope this makes sense.
Solution 4
= f.input_field :title, as: :hidden, value: "some value"
Is also an option. Note, however, that it skips any wrapper defined for your form builder.
Related videos on Youtube
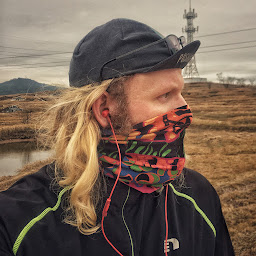
Linus Oleander
I'm a developer, student, long distance triathlete and adrenaline junkie from Gothenburg, Sweden. I'm currently doing master of science in algorithms, logic and languages at Chalmers university. Beside school I'm the founder of radiofy.se and co-founder of hprovet.se
Updated on November 02, 2020Comments
-
Linus Oleander over 3 years
How can you have a hidden field with simple form?
The following code:
= simple_form_for @movie do |f| = f.hidden :title, "some value" = f.button :submit
results in this error:
undefined method `hidden' for #SimpleForm::FormBuilder:0x000001042b7cd0
-
Linus Oleander about 13 yearsThanks, that worked.
= f.input :title, :as => :hidden, :input_html => { :value => "some value" }
-
scarver2 over 9 yearsThis is the
simple_form
way to do hidden inputs, however, if only a hidden input is needed, then just use Rails'hidden_field
form builder since Simple Form inherits all the form builder methods. -
mirap over 8 yearsOr <%= f.hidden_field :term_id, :value => @transaction.term_id %>
-
Greg Blass about 7 yearsThis should be the accepted answer. Even though the question asked about simple form, there is no reason to use it when this accomplishes the exact same thing with a shorter syntax.
-
Kiry Meas almost 6 yearsFor
simple_form_for
, thehidden
method using here is going to raise an errorundefined method
hidden' for #<SimpleForm::FormBuilder:0x00007ffa6cde0be8>`