React component function returning JSX causes error when used in render method of ES6 class React component
You are returning a react component, not a react element. The difference is an element describes how to create the component, where as the component is the instanced class. You need to return React.createElement(Struct);
, or return <Struct/>
. Also, your locals
variable will actually be a props
object. So your code should look like this:
function struct({locals}) {
return (
<View style={fieldsetStyle}>
{label}
{error}
{rows}
</View>
);
}
class Component extends React.Component {
render() {
const locals = this.getLocals();
return <Struct locals={locals}/>;
}
}
Related videos on Youtube
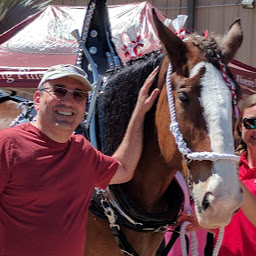
Tom Athens
Updated on June 04, 2022Comments
-
Tom Athens almost 2 years
I have a function that returns JSX as shown below:
function struct(locals) { return ( <View style={fieldsetStyle}> {label} {error} {rows} </View> ); } label, error, and rows are functions that also return JSX.
I call this function within the render method of an ES6 class component
class Component extends React.Component { render() { const locals = this.getLocals(); return struct(locals); } }
When I run the code, I get error
Element type is invalid: expected a string (for built-in components) or a class/function (for composite components) but got: object. Check the render method of
Struct
How can I get the result from struct function to render as return value of Component class render?
-
Rafael Quintanilha over 6 yearsDo you have a good reason for having this as a separate function? Why you don't turn it into a
LocalsView
component and render it passinglocals
as prop?
-