React: Fetch API getting 415 Unsupported Media Type using POST
Solution 1
It's definitely caused by the combination of mode: no-cors
and your server's cors
policy.
By using mode: no-cors
, the only headers you can use are simple headers, aka "CORS-safelisted request-headers", which only consist of application/x-www-form-urlencoded
, multipart/form-data
, or text/plain
.
This behaviour is documented here:
no-cors — Prevents the method from being anything other than HEAD, GET or POST, and the headers from being anything other than simple headers. If any ServiceWorkers intercept these requests, they may not add or override any headers except for those that are simple headers. In addition, JavaScript may not access any properties of the resulting Response. This ensures that ServiceWorkers do not affect the semantics of the Web and prevents security and privacy issues arising from leaking data across domains.
So I would:
- Remove
mode: no-cors
from your request - Check your server's allowed origins to ensure that requests from your browser's IP are allowed (for instance, if your react app is running on
http://localhost:3000
, make sure thathttp://localhost:3000
is explicitly listed as an allowed origin.
Solution 2
fetch('https://mywebapi.net/api/event/', {
method: 'POST',
headers: {
'Accept': 'application/json, text/plain',
'Content-Type': 'application/json;charset=UTF-8'
},
mode: 'no-cors',
body: {
"eventId": 5,
"eventName": "Event 5",
"eventDescription": "Event 5 Description",
"eventLocation": "Kuala Lumpur, Malaysia",
"eventDateTime": "2019-03-28"
},
}).then(response => response.text())
.then(data => console.log(data))
.catch(error => console.log("Error detected: " + error))
try it without stringifying the JSON object, as it will convert it to a string you can send the JSON object itself without converting it to a string. BTW I think you don't need to enable CORS with each request if we proxy the requests in the react app. you can enable proxy by adding this line in the package.json file.
,
"proxy": "http://localhost:5000"
assuming that your backend runs on localhost and on port 5000.
Related videos on Youtube
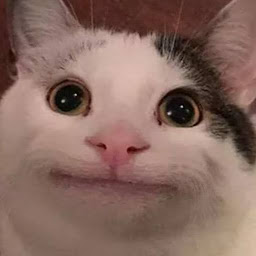
Comments
-
Chuah Cheng Jun almost 2 years
I am trying to save my data through calling my own API by using Fetch API. But then the result is keep coming back
415 Unsupported Media Type
.Client side is using React JS with .NET Core MVC. Server side is using .NET Core Web API hosted on Windows Server 2012.
I've tried all the solutions provided in the net but then I still getting 415 error. On the IIS side, I've added
Content-Type
andAccept
to acceptapplication/json
andtext/plain
.So far only GET method works. PUT, POST, DELETE all doesn't work.
Below is my code on the client side.
fetch('https://mywebapi.net/api/event/', { method: 'POST', headers: { 'Accept': 'application/json, text/plain', 'Content-Type': 'application/json;charset=UTF-8' }, mode: 'no-cors', body: JSON.stringify({ eventId: 5, eventName: "Event 5", eventDescription: "Event 5 Description", eventLocation: "Kuala Lumpur, Malaysia", eventDateTime: "2019-03-28" }), }).then(response => response.text()) .then(data => console.log(data)) .catch(error => console.log("Error detected: " + error))
If I remove
mode: 'no-cors'
it would return 500 Internal Server Error instead.I've tried using .NET using RestSharp and it able to POST normally, but not in ReactJS. So I assumed server side configuration should be correct, but not on client side.
-
Priyamal about 5 yearshave you tried it without the
headers
section -
Chuah Cheng Jun about 5 years@Priyamal yes, it returns 415 too.
-
-
Chuah Cheng Jun about 5 yearsIf I'm not wrong, the usage of
proxy
is meant for development server only, am I correct? No, both of my web client and web API is hosted on production server already and having a valid URL, not localhost. On a side note, the code that you provide throws an error after I removeJSON. stringfy()
. It saysTypes of property 'body' are incompatible. Type '{ <body value> }' is not assignable to type 'string | FormData | Blob | ArrayBufferView | ArrayBuffer | URLSearchParams | ReadableStream<Uint8Array> | null | undefined'.