React JS Get Sum of Numbers in Array
14,306
Solution 1
You can use the reduce
:
data.reduce((a,v) => a = a + v.prix , 0 )
const data = [
{title : "One",prix:100},
{title : "Two",prix:200},
{title : "Three",prix:300}
]
console.log((data.reduce((a,v) => a = a + v.prix , 0 )))
Solution 2
The reduce() method executes a reducer function (that you provide) on each element of the array, resulting in single output value.
arr.reduce(callback, initialValue);
reducer will only return one value and one value only hence the name reduce. Callback is a function to be run for each element in the array.
and Function arguments function(total,currentValue, index,arr):
Argument Description
total Required.The initialValue, or the previously returned value of the function
currentValue Required.The value of the current element
currentIndex Optional.The array index of the current element
arr Optional.The array object the current element belongs to
in this example use this code:
const data = [
{title:"One",prix:100},
{title:"Two",prix:200},
{title:"Three",prix:300}
];
const result = data.reduce((total, currentValue) => total = total + currentValue.prix,0);
console.log(result); // 600
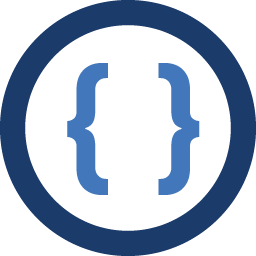
Author by
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
I have this array
const data = [ {"One",prix:100}, {"Two",prix:200}, {"Three",prix:300} ]
and I want to get the sum of all those
prix
like this:sum = 600