React-native Access Device Camera? (Android Specifically)
Solution 1
Hey @mraaron i've just made a react native app in which i have to make and upload videos, basically u can use 2 approaches:
1) React Native Image Picker https://github.com/react-community/react-native-image-picker which opens up the native camera to record videos and in response will give u the path and other info. This module has both functionality of images and videos while defining options u can specify mediaType: 'photo', 'video', or 'mixed' on iOS, 'photo' or 'video' on Android
.
2) React Native Camera https://github.com/react-native-community/react-native-camera in this u can customise the camera window as it does not open the native camera app
Note:- I have implemented both the packages and both are working absolutely fine in android as well as ios, if u need any help u can ping me up.
Solution 2
This is my demo i just made yesterday... if its helpful:
import React from 'react';
import { View, Text, Alert } from 'react-native';
import { BarCodeScanner, Permissions } from 'expo';
class CameraForm extends React.Component {
state = {
hasCameraPermission: null
};
componentDidMount() {
this.permissionCheck();
}
permissionCheck = async () => {
const { status } = await Permissions.askAsync(Permissions.CAMERA);
this.setState({
hasCameraPermission: status === 'granted'
});
};
handleBarCodeScanRead = data => {
Alert.alert(
'Scan successful!',
JSON.stringify(data)
);
};
render() {
return (
<View style={styles.container}>
<Text>Scan your wallet code</Text>
{ this.state.hasCameraPermission === null ?
<Text>Requesting for camera permission</Text> :
this.state.hasCameraPermission === false ?
<Text>Camera permission is not granted</Text> :
<BarCodeScanner
onBarCodeRead={this.handleBarCodeScanRead}
style={{ height: 400, width: 400, marginTop: 20 }}
/>
}
</View>
);
}
}
const styles = {
container: {
flex: 1,
alignSelf: 'stretch',
alignItems: 'center',
justifyContent: 'center',
backgroundColor: 'white'
}
};
export default CameraForm
;
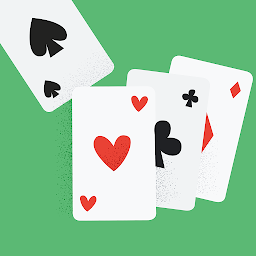
Z_z_Z
Updated on June 15, 2022Comments
-
Z_z_Z about 2 years
I'm trying to allow users to upload a video in a react-native application, but am having trouble even getting access to the camera.
** Not using Expo. I used the react-native cli and used react-native-init to generate my project **
** Using react-native version 0.53.0. Android version 5.1 **
** UPDATE: After some good advice, I've changed the compileSdkVersions and targetSdkVersions in my android/app/build.gradle file to > 23 and know that the problem isn't with my permissions. Also, the deprecated RCTCamera version of react-native-camera works fine and I'm able to capture still images and video and save them to the device. Only the master version, which uses RNCamera, still crashes the app every time the screen loads :/
This is less than ideal because I don't want to have deprecated code in my app. So when I'm referring to react-native-camera below, I mean the current version that uses RNCamera and not RCTCamera. **
I tried using the react-native-camera package (https://github.com/react-native-community/react-native-camera) but this causes my app to crash every time. Plus I want to use a camera app that the user has already installed, rather than having to build my own camera view, which is what react-native-camera requires.
Looking around, I have stumbled across three promising ways to solve this:
1) Linking - As far as I understand from this StackOverflow post (React native send a message to specific whatsapp Number2) linking can be used to open other apps the user has on their device. I figure that this can be used to access camera apps as well. But I haven't found any info on this. How do I check that a user has a camera app, and then link to them? Ideally a pop-up menu would appear on the user's phone asking the user to choose from a list of available camera apps to use.
2) This post from the android developer's docs - https://developer.android.com/training/camera/videobasics.html . This describes how to do exactly what I want to do, but I am having trouble making a native module for use in my components. I have very basic knowledge of building bridges in react-native, and was only able to make a simple native Toast module work after reading a couple articles laying out all the code. So could anyone write a VideoModule.java file that can be used to implement the same functionality as the android docs specify? This seems like the easiest solution to me, but my lack of knowledge of Java/android is standing in the way.
3) ReactNativeWebRTC - I have already included this module (https://github.com/oney/react-native-webrtc) successfully on a different screen in the application. But as I am using this module to stream video between two peers, it don't see how to use it to upload video. I looked to see if there was something akin to the MediaRecorder API that I am using for the web version of the app, but I haven't had any luck. But I know that if I can get the binary data from the media stream, then I can send this directly to my server. So, is there a way to directly store the media streams from the getUserMedia() method that react-native-webrtc employs in a buffer without a MediaRecorder like on the web?
Any solution would be tremendously helpful here. And since I only have an Android phone to test on currently, I don't need info for how to make this work with iOS. Just a solution for android. Thank you very much.
Here's my AndroidManifest.xml permissions:
<uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW"/> <uses-permission android:name="android.permission.CAMERA" /> <uses-feature android:name="android.hardware.camera" /> <uses-feature android:name="android.hardware.camera.autofocus"/> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/> <uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" /> <uses-permission android:name="android.permission.RECORD_AUDIO" /> <uses-permission android:name="android.permission.RECORD_VIDEO" /> <uses-permission android:name="android.permission.WAKE_LOCK" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
My android/app/build.gradle file:
apply plugin: "com.android.application" import com.android.build.OutputFile /** * The react.gradle file registers a task for each build variant (e.g. bundleDebugJsAndAssets * and bundleReleaseJsAndAssets). * These basically call `react-native bundle` with the correct arguments during the Android build * cycle. By default, bundleDebugJsAndAssets is skipped, as in debug/dev mode we prefer to load the * bundle directly from the development server. Below you can see all the possible configurations * and their defaults. If you decide to add a configuration block, make sure to add it before the * `apply from: "../../node_modules/react-native/react.gradle"` line. * * project.ext.react = [ * // the name of the generated asset file containing your JS bundle * bundleAssetName: "index.android.bundle", * * // the entry file for bundle generation * entryFile: "index.android.js", * * // whether to bundle JS and assets in debug mode * bundleInDebug: false, * * // whether to bundle JS and assets in release mode * bundleInRelease: true, * * // whether to bundle JS and assets in another build variant (if configured). * // See http://tools.android.com/tech-docs/new-build-system/user-guide#TOC-Build-Variants * // The configuration property can be in the following formats * // 'bundleIn${productFlavor}${buildType}' * // 'bundleIn${buildType}' * // bundleInFreeDebug: true, * // bundleInPaidRelease: true, * // bundleInBeta: true, * * // whether to disable dev mode in custom build variants (by default only disabled in release) * // for example: to disable dev mode in the staging build type (if configured) * devDisabledInStaging: true, * // The configuration property can be in the following formats * // 'devDisabledIn${productFlavor}${buildType}' * // 'devDisabledIn${buildType}' * * // the root of your project, i.e. where "package.json" lives * root: "../../", * * // where to put the JS bundle asset in debug mode * jsBundleDirDebug: "$buildDir/intermediates/assets/debug", * * // where to put the JS bundle asset in release mode * jsBundleDirRelease: "$buildDir/intermediates/assets/release", * * // where to put drawable resources / React Native assets, e.g. the ones you use via * // require('./image.png')), in debug mode * resourcesDirDebug: "$buildDir/intermediates/res/merged/debug", * * // where to put drawable resources / React Native assets, e.g. the ones you use via * // require('./image.png')), in release mode * resourcesDirRelease: "$buildDir/intermediates/res/merged/release", * * // by default the gradle tasks are skipped if none of the JS files or assets change; this means * // that we don't look at files in android/ or ios/ to determine whether the tasks are up to * // date; if you have any other folders that you want to ignore for performance reasons (gradle * // indexes the entire tree), add them here. Alternatively, if you have JS files in android/ * // for example, you might want to remove it from here. * inputExcludes: ["android/**", "ios/**"], * * // override which node gets called and with what additional arguments * nodeExecutableAndArgs: ["node"], * * // supply additional arguments to the packager * extraPackagerArgs: [] * ] */ project.ext.react = [ entryFile: "index.js" ] apply from: "../../node_modules/react-native/react.gradle" /** * Set this to true to create two separate APKs instead of one: * - An APK that only works on ARM devices * - An APK that only works on x86 devices * The advantage is the size of the APK is reduced by about 4MB. * Upload all the APKs to the Play Store and people will download * the correct one based on the CPU architecture of their device. */ def enableSeparateBuildPerCPUArchitecture = false /** * Run Proguard to shrink the Java bytecode in release builds. */ def enableProguardInReleaseBuilds = false android { compileSdkVersion 26 buildToolsVersion "25.0.2" defaultConfig { applicationId "com.slimnative" minSdkVersion 16 targetSdkVersion 26 versionCode 1 versionName "1.0" ndk { abiFilters "armeabi-v7a", "x86" } } splits { abi { reset() enable enableSeparateBuildPerCPUArchitecture universalApk false // If true, also generate a universal APK include "armeabi-v7a", "x86" } } buildTypes { release { minifyEnabled enableProguardInReleaseBuilds proguardFiles getDefaultProguardFile("proguard-android.txt"), "proguard-rules.pro" } } // applicationVariants are e.g. debug, release applicationVariants.all { variant -> variant.outputs.each { output -> // For each separate APK per architecture, set a unique version code as described here: // http://tools.android.com/tech-docs/new-build-system/user-guide/apk-splits def versionCodes = ["armeabi-v7a":1, "x86":2] def abi = output.getFilter(OutputFile.ABI) if (abi != null) { // null for the universal-debug, universal-release variants output.versionCodeOverride = versionCodes.get(abi) * 1048576 + defaultConfig.versionCode } } } } dependencies { compile fileTree(dir: "libs", include: ["*.jar"]) compile "com.android.support:appcompat-v7:23.0.1" compile "com.facebook.react:react-native:+" // From node_modules compile project(':WebRTCModule') compile project(':react-native-svg') compile (project(':react-native-camera')) { // exclude group: "com.google.android.gms" exclude group: "com.android.support" } // compile ("com.google.android.gms:play-services-vision:10.2.0") { // force = true; // } compile ('com.android.support:exifinterface:26.0.1') { force = true; } } // Run this once to be able to run the application with BUCK // puts all compile dependencies into folder libs for BUCK to use task copyDownloadableDepsToLibs(type: Copy) { from configurations.compile into 'libs' }
And my android/build.gradle:
buildscript { repositories { jcenter() } dependencies { classpath 'com.android.tools.build:gradle:2.2.3' // NOTE: Do not place your application dependencies here; they belong // in the individual module build.gradle files } } allprojects { repositories { mavenLocal() jcenter() maven { // All of React Native (JS, Obj-C sources, Android binaries) is installed from npm url "$rootDir/../node_modules/react-native/android" } maven { url "https://jitpack.io" } maven { url "https://maven.google.com" } } }
-
Sagar Chavada over 6 yearsfirst tell me R you running app with expo or what? and have you ask for runtime camera permission in android
-
Z_z_Z over 6 years@SagarChavada I am not running expo. I used react-native cli and used react-native init to create my app. And I tried copy/paste the example from react-native-camera directly (github.com/react-native-community/react-native-camera/tree/…), but the app crashed as soon as I hit the upload page. There were no requests to access my camera anywhere, even though I added all permissions. Strangely, I never got a prompt to give permission to my camera when using the react-native-webrtc module, though that is working perfectly. So maybe this module is short-circuiting permissions?
-
Sagar Chavada over 6 years
-
Z_z_Z over 6 years@SagarChavada Already tried using PermissionsAndroid as well. I still didn't get any requests for permissions. Instead, I got a blank black screen
-
Sagar Chavada over 6 yearsok.. just post your .gradle code, and always mention react-native version in question...
-
Z_z_Z over 6 years@SagarChavada updated to put my version, which is 0.53.0 and I included the .gradle files
-
Sagar Chavada over 6 yearsbro.. not just dependency..
-
Z_z_Z over 6 years@SagarChavada Ok, I'll update to show the whole file
-
Z_z_Z over 6 years@SagarChavada Posted the full file. Sorry if the spacing is a bit off
-
Sagar Chavada over 6 yearsok.. just change it to 25,and run again with your permission code
-
Z_z_Z over 6 years@SagarChavada change what exactly to 25?
-
Sagar Chavada over 6 yearsyour targetSDKVersion, compileSDkVersion, buildtoolversion, afterward clean build and cache and run it again..
-
Z_z_Z over 6 years@SagarChavada I changed my compileSdkVersion and targetSdkVersion to 25. I also upgraded my build tools to 27.0.3 and set them accordingly. I will try cleaning the cache as well. Changing the Sdkversion definitely helped with my permissions. But the master version of react-native-camera still crashes. However, the deprecated RCTCamera works now and I can capture a still image and video just fine
-
Sagar Chavada over 6 yearsi m glad its work now, and for native-camera you should follow its github and track issue there? think like a developer..
-
-
Z_z_Z over 6 yearsYes, I have checked all my permissions in the androidmanifest.xml file. I will add my file to my question so you can take a look. And I want to use an external camera so that the user has a greater sense of comfortability since they're probably used to using that app all the time. But it is not essential to have it be external
-
Suhail Jain over 6 yearsit'd be great if you could upload android manifest, i would recommend using the react-native-camera view as then you would be in control of what you think your application's camera view should look like, saying that, what i mean is say for example you are designing this app to just read barcodes from your camera view then using your custom view you can render a simple view avoiding things like faceDetection, picking image from gallery etc etc.
-
Z_z_Z over 6 yearsI added my manifest file just now. Thanks for the recommendation, I will take that in consideration. Hopefully, I just have some stupid typo somewhere and I can use the react-native-camera
-
Suhail Jain over 6 yearsi hope you are not using the camera view in 'front' mode because i do not see the permission for using front-camera
-
Sagar Chavada over 6 yearsdont warry.. there is no permission like front camera... just need to access camera ..
-
Suhail Jain over 6 years@SagarChavada there is a dedicated permission , <uses-feature android:name="android.hardware.camera.front" android:required="false"/>
-
Sagar Chavada over 6 yearsi m saying that its not require to add.. bcz by-default its enable as front.. but if you want to disable than its needed
-
Suhail Jain over 6 yearsplease go through this doc developer.android.com/guide/topics/manifest/… for getting clarity on different camera permissions on android and there inter-dependencies
-
Z_z_Z over 6 yearsI will try and use your permissions model in my app. Thanks for sharing this
-
Z_z_Z over 6 yearsOn the react-native-camera docs, there is no mention of adding <uses-feature android:name="android.hardware.camera.front" android:required="false"/> , as far as I can tell anyways... But I will read the doc you provided and try adding the line to my code to see what happens
-
Suhail Jain over 6 yearscheers! @SagarChavada. mraaron, yes i think it is worthy enough to give a try, lets see if you get through.
-
Z_z_Z over 6 yearsThanks for the info! I will try out the react-native-image-picker later today! Though from the quick glance I did now, this seems to be a much better solution to my problem
-
Z_z_Z over 6 yearsAfter testing it out, I think that the react-native-image-picker is the best choice here. You saved me a lot of headaches here
-
Nunchucks about 6 yearsPSA. I've found react-native-image-picker to be buggy on Android. Could be something unique to my case, but it works perfectly until you try to change a photo for the second time at which point it crashes and restarts the app. The request still goes through and updates the photo though. ¯_(ツ)_/¯ "react-native-image-picker": "^0.26.10"; "react-native": "0.51.0",
-
Niloufar over 4 yearsThanks for your info, I want to know is there any way to have both video and image on Android as well?