ReactJs - Fetch a quote every 5 seconds with axios and display it in the <p>
10,566
You could do something like the following.
You should use the componentDidMount
lifecycle method to make the fetch request. You can also use setInterval
there too to trigger the api request every 5 seconds (5000 ms). In the componentWillUnmount
section you should clear the interval.
class App extends React.Component {
state = {
joke: ""
};
componentDidMount() {
this.getJoke();
this.interval = setInterval(() => {
this.getJoke();
}, 5000);
}
getJoke() {
fetch("https://api.chucknorris.io/jokes/random")
.then(res => {
return res.json();
})
.then(res => {
this.setState({
joke: res.value
});
});
}
componentWillUnmount() {
clearInterval(this.interval);
}
render() {
return <p>{this.state.joke}</p>;
}
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.1.0/react-dom.min.js"></script>
<div id="root"></div>
Related videos on Youtube
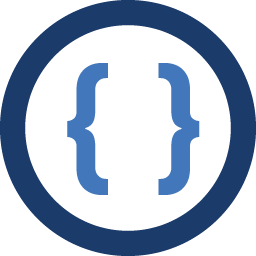
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am new to Reactjs programming and I have a component which uses Grid of react bootstrap.
I want to fetch a quoute from some public API every 5 seconds and display it in the
inside the Grid. I also want to use axios for fetching the quote. Any tutorial or any help would be appreciated. Thank you :)
-
Tholle almost 6 yearsWe'd like to help you, but this question is very broad. Please include what you have tried so far, and read How do I ask a good question.
-