Read binary file, save in buffer, print out content of buffer
Recommended changes:
Change your buffer to a
char
(byte), as theftell()
will report the size in bytes (char
) andmalloc()
use the byte size also.unsigned int buffer []; /buffer/
to
unsigned char *buffer; /*buffer*/
[Edit] 2021: Omit cast
2) This is OK, size is bytes and buffer points to bytes, but could be explicitly castbuffer = malloc(size); /allocate space on heap/
to
buffer = (unsigned char *) malloc(size); /*allocate space on heap*/
/* or for those who recommend no casting on malloc() */
buffer = malloc(size); /*allocate space on heap*/
change 2nd parameter from
sizeof(unsigned int)
tosizeof *buffer
, which is 1.else if (fread(buffer, sizeof(unsigned int), size, fp) != size){
to
else if (fread(buffer, sizeof *buffer, size, fp) != size){
Change
"%x"
to"%02x"
else single digit hexadecimal numbers will confuse the output. E. g. is "1234" four bytes or two?printf("%x", buffer[i]);
to
printf("%02x", buffer[i]);
Your clean-up at the end of the function may include
fclose(fp); free(buffer);
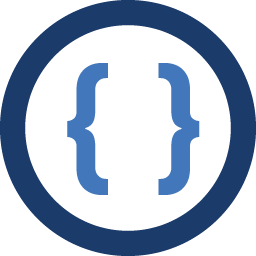
Admin
Updated on March 09, 2021Comments
-
Admin about 3 years
I have a big problem that need's to be solved before I can continue with my program.
I have to open a binary file, read it's content, save the content into a buffer, allocate space on the heap with malloc, close the file and finally printf( the content of the .bin file). I came this far (closing file is not implemented yet):
void executeFile(char *path){ FILE *fp; /*filepointer*/ size_t size; /*filesize*/ unsigned int buffer []; /*buffer*/ fp = fopen(path,"rb"); /*open file*/ fseek(fp, 0, SEEK_END); size = ftell(fp); /*calc the size needed*/ fseek(fp, 0, SEEK_SET); buffer = malloc(size); /*allocalte space on heap*/ if (fp == NULL){ /*ERROR detection if file == empty*/ printf("Error: There was an Error reading the file %s \n", path); exit(1); } else if (fread(&buffer, sizeof(unsigned int), size, fp) != size){ /* if count of read bytes != calculated size of .bin file -> ERROR*/ printf("Error: There was an Error reading the file %s - %d\n", path, r); exit(1); }else{int i; for(i=0; i<size;i++){ printf("%x", buffer[i]); } } }
I think I messed up the buffer and I am not really sure if I read the .bin file correctly because I can't print it with
printf("%x", buffer[i])
Hope you guys can help
Greetings from germany :)
-
Jonathan Leffler almost 11 yearsYou can't use an empty array bound like that inside a function (OK in an argument list). And you can't assign to an array like the
malloc()
does. It needs to beunsigned int *buffer;
. -
Admin almost 11 yearsyes he is complaining about the empty array bound ... the program does not know how big the buffer has to be because it doesn't know what size the file has ...
-
chux - Reinstate Monica almost 11 years@Jonathan Leffler : corrected solution from "unsigned int buffer[]" to "unsigned char *buffer".
-
chux - Reinstate Monica over 7 years@luizfls Why yes it is! Code amended.