Read int values from a text file in C
218,906
Solution 1
A simple solution using fscanf
:
void read_ints (const char* file_name)
{
FILE* file = fopen (file_name, "r");
int i = 0;
fscanf (file, "%d", &i);
while (!feof (file))
{
printf ("%d ", i);
fscanf (file, "%d", &i);
}
fclose (file);
}
Solution 2
How about this?
fscanf(file,"%d %d %d %d %d %d %d",&line1_1,&line1_2, &line1_3, &line2_1, &line2_2, &line3_1, &line3_2);
In this case spaces in fscanf
match multiple occurrences of any whitespace until the next token in found.
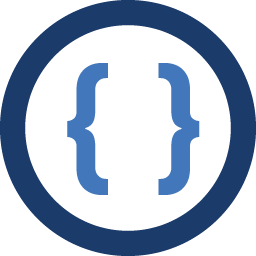
Author by
Admin
Updated on June 08, 2020Comments
-
Admin almost 4 years
I have a text file that contains the following three lines:
12 5 6 4 2 7 9
I can use the
fscanf
function to read the first 3 values and store them in 3 variables. But I can't read the rest. I tried using thefseek
function, but it works only on binary files.Please help me store all the values in integer variables.