Reading a pfx file from usb token with java
Solution 1
The whole point of a USB token for signing is, that nobody can read the secret key from that device. So you sent the hash to the token and the token will send you the signature back.
For this to work you need a JCE provider which can talk to the token. This is typically done either by PKCS#11 (the token delivers a library for this) or the token delivers a MSCAPI driver (under windows).
Both can be used under Java, the PKCS#11 way might be a bit more complicated to setup, but in my experience it is better for automated signing because in the MSCAPI case you often need to enter the token PIN manually.
If your token is recognized by windows the following command should see and list its key:
keytool -list -storetype Windows-MY
The Windows Keystore can then be used to get a handle of the key for signing, but you can also use it to export a copy of the public key.
Solution 2
You can use SUN PKCS11 provider to refer the keys in the Etoken.You can just try the below code
String pkcs11Config = "name=eToken\nlibrary=C:\\Windows\\System32\\eps2003csp11.dll";
java.io.ByteArrayInputStream pkcs11ConfigStream = new java.io.ByteArrayInputStream(pkcs11Config.getBytes());
sun.security.pkcs11.SunPKCS11 providerPKCS11 = new sun.security.pkcs11.SunPKCS11("pkcs11Config");
java.security.Security.addProvider(providerPKCS11);
// Get provider KeyStore and login with PIN
String pin = "12345678";
java.security.KeyStore keyStore = java.security.KeyStore.getInstance("PKCS11", providerPKCS11);
KeyStore keyStore=KeyStore.getInstance("PKCS11",providerPKCS11);
keyStore.load(null, pin.toCharArray());
// Enumerate items (certificates and private keys) in the KeyStore
java.util.Enumeration<String> aliases = keyStore.aliases();
String alias = null;
while (aliases.hasMoreElements()) {
alias = aliases.nextElement();
System.out.println(alias);
}
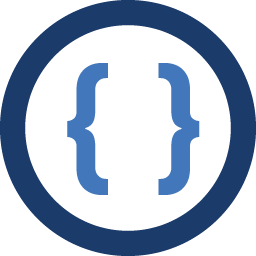
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I am trying to sign a pdf document in java using a USB e-token.I want to read the signature from USB token safenet (alladin etoken pro 72 k(Java)) and attach to pdf using java code.I have done digital signature signing using a key stored in my local machine.But i want to know how the same can be done using a USB e-token.
-
Prashant over 8 yearsThis asumes a file input not a token.
-
hadi.mansouri over 5 yearsThe answer is not about tokens. It is about work with keystore files with format PKCS12.