Reading email from gmail is not working
Change Your emailSession
variable with
Session emailSession = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(userName, password);
}
});
As said by Gmail
Some apps and devices use less secure sign-in technology, which makes your account more vulnerable. You can turn off access for these apps, which we recommend, or turn on access if you want to use them despite the risks. Learn more
Just click below link and disable Gmail security settings.It will work.
Here is a nice article about Password Authentication in Java Mail Api
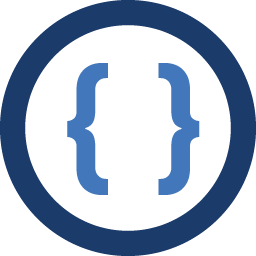
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
I am using Java Mail API along with the following code to read email from my gmail account.
import java.util.Properties; import javax.mail.Folder; import javax.mail.Message; import javax.mail.MessagingException; import javax.mail.NoSuchProviderException; import javax.mail.Session; import javax.mail.Store; public class CheckMails { public static void check(String host, String storeType, String user, String password) { try { Properties properties = new Properties(); properties.put("mail.pop3.host", host); properties.put("mail.pop3.port", "995"); properties.put("mail.pop3.starttls.enable", "true"); Session emailSession = Session.getDefaultInstance(properties); //create the POP3 store object and connect with the pop server Store store = emailSession.getStore("pop3s"); store.connect(host, user, password); //create the folder object and open it Folder emailFolder = store.getFolder("INBOX"); emailFolder.open(Folder.READ_ONLY); // retrieve the messages from the folder in an array and print it Message[] messages = emailFolder.getMessages(); System.out.println("messages.length---" + messages.length); for (int i = 0, n = messages.length; i < n; i++) { Message message = messages[i]; System.out.println("---------------------------------"); System.out.println("Email Number " + (i + 1)); System.out.println("Subject: " + message.getSubject()); System.out.println("From: " + message.getFrom()[0]); System.out.println("Text: " + message.getContent().toString()); } //close the store and folder objects emailFolder.close(false); store.close(); } catch (NoSuchProviderException e) { e.printStackTrace(); } catch (MessagingException e) { e.printStackTrace(); } catch (Exception e) { e.printStackTrace(); } } public static void main(String[] args) { String host = "pop.gmail.com";// change accordingly String mailStoreType = "pop3"; String username = "[email protected]";// change accordingly String password = "*******";// change accordingly check(host, mailStoreType, username, password); } }
But I am getting the following exceptions:
javax.mail.AuthenticationFailedException: [AUTH] Web login required: ` https://support.google.com/mail/bin/answer.py?answer=78754` at com.sun.mail.pop3.POP3Store.protocolConnect(POP3Store.java:209) at javax.mail.Service.connect(Service.java:364) at javax.mail.Service.connect(Service.java:245) at CheckMails.check(CheckMails.java:26) at CheckMails.main(CheckMails.java:66)
But i have recieved an email from gmail in my inbox which is saying that "We recently blocked-in a sign in attempt to your Google Account". How can i make the program to work properly?