Reading file from serial port in Java
Solution 1
Have you tested with a Serial Port Emulator software?
When I did this kind of app for college, our professor told us to build the app and test it using an emulator, since it's much cheaper and less error prone.
While searching for google you can find some softwares that do that. I don't remember exactly the one we used at that time, but it worked quite well.
I mean products like this: Eterlogic - Virtual Serial Ports Emulator, but that's just an example (and I haven't tested this software, I just googled it)
Solution 2
You are erroneously assuming that full messages are always going to be received. Instead, when a serial event is triggered, only part of the message may be available. For example, you may get an event and read "FLAS" and a subsequent event will give "H_OK". You need to adapt your code to something like this:
// member variables
byte [] receiveBuffer = new byte[BUFFER_LENGTH];
int receiveIndex = 0;
// Receive code
receiveIndex +=
inputStream.read(receiveBuffer, receiveIndex, BUFFER_LENGTH - receiveIndex);
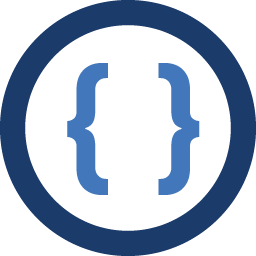
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
i'm beginner in java technology, I have to read file from port. Frst I'll write "FLASH" to outputstream then I'll get response as a "FLASH_OK" from target device, after getting FLASH_OK as response then again i have to write name of the file which i want,but problem is its not writing file name to outputstream, below is my code. Please help me.
package writeToPort; import java.awt.Toolkit; import java.io.*; import java.util.*; import javax.comm.*; import javax.swing.JOptionPane; import constants.Constants; public class Flashwriter implements SerialPortEventListener { Enumeration portList; CommPortIdentifier portId; String messageString = "\r\nFLASH\r\n"; SerialPort serialPort; OutputStream outputStream; InputStream inputStream; Thread readThread; String one, two; String test = "ONLINE"; String[] dispArray = new String[1]; int i = 0; byte[] readBufferArray; int numBytes; String response; FileOutputStream out; final int FLASH = 1, FILENAME = 2; int number; File winFile; public static void main(String[] args) throws IOException { Flashwriter sm = new Flashwriter(); sm.FlashWriteMethod(); } public void FlashWriteMethod() throws IOException { portList = CommPortIdentifier.getPortIdentifiers(); winFile = new File("D:\\testing\\out.FLS"); while (portList.hasMoreElements()) { portId = (CommPortIdentifier) portList.nextElement(); if (portId.getPortType() == CommPortIdentifier.PORT_SERIAL) { if (portId.getName().equals("COM2")) { try { serialPort = (SerialPort) portId.open("SimpleWriteApp", 1000); } catch (PortInUseException e) { } try { inputStream = serialPort.getInputStream(); System.out.println(" Input Stream... " + inputStream); } catch (IOException e) { System.out.println("IO Exception"); } try { serialPort.addEventListener(this); } catch (TooManyListenersException e) { System.out.println("Tooo many Listener exception"); } serialPort.notifyOnDataAvailable(true); try { outputStream = serialPort.getOutputStream(); inputStream = serialPort.getInputStream(); } catch (IOException e) { } try { serialPort.setSerialPortParams(9600, SerialPort.DATABITS_8, SerialPort.STOPBITS_1, SerialPort.PARITY_NONE); serialPort .setFlowControlMode(SerialPort.FLOWCONTROL_NONE); number = FLASH; sendRequest(number); } catch (UnsupportedCommOperationException e) { } } } } } public void serialEvent(SerialPortEvent event) { SerialPort port = (SerialPort) event.getSource(); switch (event.getEventType()) { case SerialPortEvent.DATA_AVAILABLE: try { if (inputStream.available() > 0) { numBytes = inputStream.available(); readBufferArray = new byte[numBytes]; int readBytes = inputStream.read(readBufferArray); one = new String(readBufferArray); System.out.println("readBytes " + one); } if (one.indexOf("FLASH_") > -1 & !(one.indexOf("FLASH_F") > -1)) { System.out.println("got message"); response = "FLASH_OK"; number = FILENAME; sendRequest(number); } out = new FileOutputStream(winFile, true); out.write(readBufferArray); out.close(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } readBufferArray = null; // break; } } public void sendRequest(int num) { switch (num) { case FLASH: try { outputStream.write(messageString.getBytes()); outputStream.flush(); } catch (IOException e) { e.printStackTrace(); } break; case FILENAME: try { outputStream.write("\r\n26-02-08.FLS\r\n".getBytes()); outputStream.flush(); } catch (IOException e) { e.printStackTrace(); } break; } } }
-
Admin about 15 yearsya i'm getting response as flash ok,but i'm unable to write to outputstream for second time.
-
Admin about 15 yearsi'm reading serailport and data will come for every one second but some time some seconds of data is missing,wat will be the problem plz suggest me
-
Edison Gustavo Muenz about 15 yearsIn that case the problem is with your hardware. Try testing it on different machines and different cables. But only do that if you have already done some extensive testing with the emulator to see if the bug is not on your code