Recursively create directory
Solution 1
public static void main(String[] args) {
File root = new File("C:\\SO");
List<String> alphabet = new ArrayList<String>();
for (int i = 0; i < 26; i++) {
alphabet.add(String.valueOf((char)('a' + i)));
}
final int depth = 3;
mkDirs(root, alphabet, depth);
}
public static void mkDirs(File root, List<String> dirs, int depth) {
if (depth == 0) return;
for (String s : dirs) {
File subdir = new File(root, s);
subdir.mkdir();
mkDirs(subdir, dirs, depth - 1);
}
}
mkDirs
recusively creates a depth
-level directory tree based on a given list of String
s, which, in the case of main
, consists of a list of characters in the English alphabet.
Solution 2
You can simply use the mkdirs() method of java.io.File
class.
Example:
new File("C:\\Directory1\\Directory2").mkdirs();
Solution 3
If you don't mind relying on a 3rd party API, the Apache Commons IO package does this directly for you. Take a look at FileUtils.ForceMkdir.
The Apache license is commercial software development friendly, i.e. it doesn't require you to distribute your source code the way the GPL does. (Which may be a good or a bad thing, depending on your point of view).
Solution 4
Since Java 7, java.nio is preferred
import java.nio.file.Files;
import java.nio.file.Paths;
...
Files.createDirectories(Paths.get("a/b/c"));
Solution 5
If you're using mkdirs()
(as suggested by @Zhile Zou) and your File
object is a file and not a directory, you can do the following:
// Create the directory structure
file.getParentFile().mkdirs();
// Create the file
file.createNewFile();
If you simply do file.mkdirs()
it will create a folder with the name of the file.
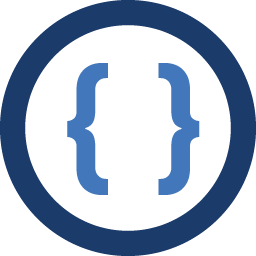
Admin
Updated on October 07, 2020Comments
-
Admin over 3 years
Does anyone know how to use Java to create sub-directories based on the alphabets (a-z) that is n levels deep?
/a /a /a /b /c .. /b /a /b .. .. /a /b /c .. /b /a /a /b .. /b /a /b .. .. /a /b .. .. /a /a /b .. /b /a /b .. .. /a /b ..