Redirect to requested page after authentication
If you create an ASP.NET MVC 3 or 4 Internet Application project, it'll have a complete example of how to use return url's when authenticating.
When you add the AuthorizeAttribute to a controller to force authentication, it'll redirect the user to your Login method, and automatically append the returnUrl parameter. From there, you need to keep track of it as you show your login form:
public ActionResult Login(string returnUrl)
{
ViewBag.ReturnUrl = returnUrl;
return View();
}
and then add it to your login form's route collection:
@*//ReSharper disable RedundantAnonymousTypePropertyName*@
@using (Html.BeginForm(new { ReturnUrl = ViewBag.ReturnUrl })) {
@*//ReSharper restore RedundantAnonymousTypePropertyName*@
}
Once the user submits the login, assuming they authenticate properly, you'll just redirect to returnUrl:
[HttpPost]
public ActionResult Login(LoginModel model, string returnUrl)
{
return RedirectToLocal(returnUrl);
}
The hardest part is keeping track of the ReturnUrl through the GET/POST sequence.
If you want to see how the AuthorizeAttribute works, this StackOverflow post shows setting returnUrl with the original request.
You also need to make sure you validate returnUrl really is a local url, or you become vulnerable to open redirection attacks. RedirectToLocal() is a helper method from the MVC 4 Internet Application template that does this validation:
private ActionResult RedirectToLocal(string returnUrl)
{
if (Url.IsLocalUrl(returnUrl))
{
return Redirect(returnUrl);
}
else
{
return RedirectToAction("Index", "Home");
}
}
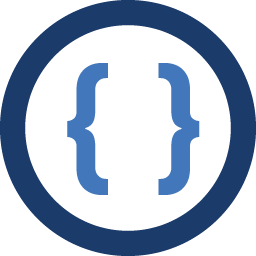
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I'm working on an mvc .net application and I'm using forms authentication. I want to redirect user to the page he requested after he gets authenticated. Any help would be appreciated.
-
mohsen dorparasti over 11 years@HediNaily : one point to remeber is not to trust returnUrl and to check it before using it to redirect the user
-
mfanto over 11 years@mohsen.d Agreed. The RedirectToLocal() is an MVC 4 helper included with the Internet Application template that validates the URL before redirecting. I'll add it to the answer for those without the helper method.
-
hvaughan3 about 7 yearsOne important thing for those ReSharper users, ReSharper will tell you that you can remove the
ReturnUrl =
part of theHtml.BeginForm
since an explicit name is not needed. In this case it is needed.