Redirection after successful form submit
Solution 1
You should bind to the submit
event, not click
event:
UPDATED TO MATCH THE COMMENTS
$(function () {
var submityesClicked;
//catch the click to buttons
$('#submityes').click(function () {
submityesClicked = true;
});
$('#submitno').click(function () {
submityesClicked = false;
});
$('#webform').submit(function (e) {
e.preventDefault();//prevent the default action
$.ajax({
type: "POST",
/*url: "process.php", //process to mail
data: $('form.contact').serialize(),*/
success: function (msg) {
window.location.replace(submityesClicked ? "/submit_resolved_yes.php" : "/submit_resolved_no.php");
},
error: function () {
alert("error");
}
});
});
});
The submit
event is triggered only if the form is valid.
Note that the submit
event is triggered by the form but the click
event is triggered by the input
element.
Solution 2
Do redirection on complete. Not on success
$('document').ready(function () {
"use strict";
$(function () {
$('#submityes').click(function () {
$.ajax({
type: "POST",
/* url: "process.php", //process to mail
data: $('form.contact').serialize(), */
success: function (msg) {
//window.location.replace("/submit_resolved.php");
},
complete: function () {
window.location.replace("/submit_resolved.php");
},
error: function () {
alert("error");
}
});
});
});
});
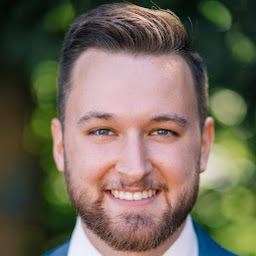
Tim Kühn
Business IT student - I'm not that good in programming so stackoverflow pretty much saves my life in university/at work! :)
Updated on June 28, 2022Comments
-
Tim Kühn almost 2 years
I have a form which should submit data after pressing the submit button. After tagging a few input fields as required the form always shows me when there is no input in the required field after pressing the submit button - so far, so good.
What I would like to realize is that there is a redirection to another page if the submission was successful. If there are some empty required fields the form should show me, without redirecting me to another page.
By now I have the following code:
Submit button:
<div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <button type="submit" name="submityes" id="submityes" class="btn btn-danger">Submit</button> </div> </div>
Also I have the following js function to submit the form and to redirect me to another page:
$('document').ready(function () { "use strict"; $(function () { $('#submityes').click(function () { $.ajax({ type: "POST", /* url: "process.php", //process to mail data: $('form.contact').serialize(), */ success: function (msg) { window.location.replace("/submit_resolved.php"); }, error: function () { alert("error"); } }); }); }); });
The problem I have right now is that I will always be redirected to the "submit_resolved.php" page, whether all required fields are complete or not.
How can I solve this problem? I only want to be redirected when all required fields are not empty.
-
nnnnnn about 7 yearsThe OP doesn't want any redirection if the form didn't validate successfully (as in, no redirection if required fields were left blank).
-
Constantin Galbenu about 7 yearsbut what happens if the form is not valid from the backend point of view?
-
Alex Slipknot about 7 yearsMaybe my misunderstanding... But anyway first we have to validate - before submit, then send request via ajax and when response received - check if all ok and process result in success. Then if all ok, in complete we have to redirect user. Am I right?
-
ebu_sho about 7 yearsthat's another question
-
Tim Kühn about 7 yearsThank you very much. Is the function isValid() the back end validation? And I guess I need to check every single required variable for this function?
-
Aravindh Gopi about 7 yearsu can do it in frontend as well as backend
-
Aravindh Gopi about 7 yearsu can use form.elemets within a for loop to validate every elements in your forms
-
Tim Kühn about 7 yearsThank you. So to bind the submit event I need to change the selector from '#submityes' to something else? Unfortunately I am not sure which expression to write in there.
-
Tim Kühn about 7 yearsOh, I think I got it. I gave the form the name/id "webform" and I wrote '#webform' instead of '#submityes' into the brackets. Now I have another little problem: the form has two different submit buttons ('#submityes' and '#submitno'). Both should redirect the user to a different page. How can I implement this into the code? It should be something like "if '#webform' is submitted via '#submityes' redirect to X, if it is submitted via '#submitno' redirect to Y'
-
Thierry Lemaitre about 4 yearsSomething that I don't understand is why should I use the preventDefault()? Because of that, my form is not sent to my email. But without the preventDefault() my form is sent and my redirection is working too. So how is it usefull to have it?
-
Constantin Galbenu about 4 yearsIf you don't prevent the default behavior of the form then it will be send two times, first in the AJAX call and second as the default behavior when a user press the submit button.