Regex: Match words in sentence PHP
Solution 1
To Match each words like "walk" but not "walking" Use \b for word bounday.
For Example, "\bwalk\b"
Solution 2
function magicWords($words, $string) {
$from = $to = array();
foreach($words as $word) {
$from[] = "/\b$word\b/i"; // \b represents a word boundary
$to[] = '<a href="#' . strtolower($word) . '">${0}</a>';
}
return preg_replace($from, $to, $string);
}
$words = array('go', 'walk');
echo magicWords($words, "Lets go walking on a Walk");
This outputs:
'Lets <a href="#go">go</a> walking on a <a href="#walk">Walk</a>.'
Note that it matches "go", and "walk", but not "walking", and maintains the capital W on Walk while the link becomes lower case "#walk".
This way, "Walk walk WALK wALk" will all link to #walk without affecting the original formatting.
Solution 3
Try something like this:
$words = array('walk','talk');
foreach($words as $word)
{
$word = preg_replace("/\b$word\b/","< a href='#$word' >$word< /a >",$word);
}
Solution 4
I think the following might be what you want.
<?php
$someText = 'I don\'t like walking, I go';
$words = array('walk', 'go');
$regex = '/\\b((' . implode('|',$words) . ')\\b(!|,|\\.|\\?)?)/i';
echo preg_replace_callback(
$regex,
function($matches) {
return '<a href=\'' . strtolower($matches[2]) . '\'>' . $matches[1] . '</a>';
},
$someText);
?>
A few of points though:
- This solution and all the others will match any occurrences of the word be they in element attributes or whatever
- I've added a bit on the end for punctuation matching should you want to include it within the link/anchor tags.
- This requires php 5.3 anonymous functions. I tought this was an interesting alternative to the foreach methods mentioned
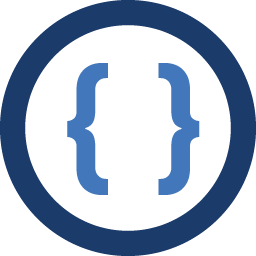
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
I have an array with words like
$arr = array("go", "walk", ...)
I would like to replace these words with links f they are matched in sentences. But it should be only if they match exactly (for example "walk" should match "Walk" or "walk!" but not also "walking")
And the replacement should be a simple link like:
< a href='#walk' >walk< /a >
Anybody Any idea?