Regular expression to split string and number
Solution 1
I know you asked for the Split
method, but as an alternative you could use named capturing groups:
var numAlpha = new Regex("(?<Alpha>[a-zA-Z]*)(?<Numeric>[0-9]*)");
var match = numAlpha.Match("codename123");
var alpha = match.Groups["Alpha"].Value;
var num = match.Groups["Numeric"].Value;
Solution 2
splitArray = Regex.Split("codename123", @"(?<=\p{L})(?=\p{N})");
will split between a Unicode letter and a Unicode digit.
Solution 3
Regex is a little heavy handed for this, if your string is always of that form. You could use
"codename123".IndexOfAny(new char[] {'1','2','3','4','5','6','7','8','9','0'})
and two calls to Substring.
Solution 4
A little verbose, but
Regex.Split( "codename123", @"(?<=[a-zA-Z])(?=\d)" );
Can you be more specific about your requirements? Maybe a few other input examples.
Solution 5
IMO, it would be a lot easier to find matches, like:
Regex.Matches("codename123", @"[a-zA-Z]+|\d+")
.Cast<Match>()
.Select(m => m.Value)
.ToArray();
rather than to use Regex.Split
.
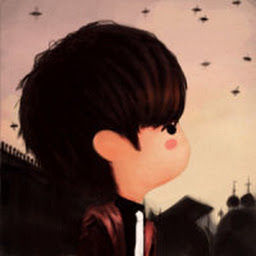
Yinda Yin
I have been a software developer for many years. I have written software for NASA, developed a Practice Management program for CPA's, and have worked at a compliance management company doing cutting-edge work in C#, WPF and Aurelia. I am a strong believer in code clarity and simplicity.
Updated on July 09, 2022Comments
-
Yinda Yin almost 2 years
I have a string of the form:
codename123
Is there a regular expression that can be used with
Regex.Split()
to split the alphabetic part and the numeric part into a two-element string array?