relative file paths in perl
Solution 1
FindBin is the classic solution to your problem. If you write
use FindBin;
then the scalar $FindBin::Bin
is the absolute path to the location of your Perl script. You can chdir
there before you open the data file, or just use it in the path to the file you want to open
my $rawDataName = "$FindBin::Bin/../dataFileToRead.txt";
open my $in, "<", $rawDataName;
(By the way, it is always better to use lexical file handles on anything but a very old perl.)
Solution 2
To turn a relative path into an absolute one you can use Cwd :
use Cwd qw(realpath);
print "'$0' is '", realpath($0), "'\n";
Solution 3
Start by finding out where the script is.
Then get the directory it is in. You can use Path::Class::File's dir()
method for this.
Finally you can use chdir
to change the current working directory to the directory you just identified.
So, in theory:
chdir(Path::Class::File->new(abs_path($0))->dir());
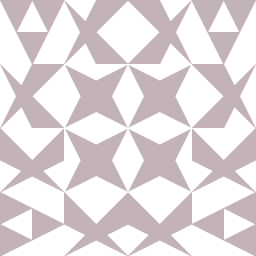
Dunc
Updated on June 04, 2022Comments
-
Dunc over 1 year
I have a perl script which is using relative file paths.
The relative paths seem to be relative to the location that the script is executed from rather than the location of the perl script. How do I make my relative paths relative to the location of the script?
For instance I have a directory structure
dataFileToRead.txt ->bin myPerlScript.pl ->output
inside the perl script I open dataFileToRead.txt using the code my $rawDataName = "../dataFileToRead.txt"; open INPUT, "<", $rawDataName;
If I run the perl script from the bin directory then it works fine
If I run it from the parent directory then it can't open the data file.
-
Borodin over 11 years@daxim: your comment is presumably the mantra that FindBin is broken. I know about this, but cannot easily find an explanation, an example of its failure relevant to this question, or an alternative module. Perhaps you can help us?
-
daxim over 11 yearsDirect links: FindBin is broken (fixed in core since mid-2011 only); FindBin seems to think it's necessary to walk the whole path; code alternative or perhaps FindBin::Real or lib::abs. I never had the desire to do this comparison because I never needed FindBin or functional equivalent because I know about packaging and the share dir feature.
-
Jean-Francois T. over 8 yearsDocumentation of Cwd is currently available here: search.cpan.org/~smueller/PathTools-3.47/Cwd.pm
-
Andy Lester over 6 yearsI've updated the path to the Cwd docs to a permanent path: search.cpan.org/dist/PathTools/Cwd.pm That path will always be the latest, even if the version changes or even the owner.