Reminder/Alarm in ionic 2
Solution 1
The plugin you are attempting to use is almost 3 years old. Older than Ionic 2 itself. You should look at the native plugins listed at https://ionicframework.com/docs/v2/native/
In the past I have used the Local Notifications plugin (https://ionicframework.com/docs/v2/native/local-notifications/) to handle reminders/alarms.
It is quite simple to schedule, all you have to do is create a Date object for the time you wish to notify. Here is an example using the data you provided:
import { LocalNotifications } from 'ionic-native';
// Schedule delayed notification
LocalNotifications.schedule({
text: 'Alarm has expired!',
at: new Date(new Date().getTime() + 3600),
sound: isAndroid ? 'file://sound.mp3': 'file://beep.caf',
data: { message : 'json containing app-specific information to be posted when alarm triggers' }
});
Solution 2
To trigger it repeatedly, you can use below code. It will trigger notification daily. Reference
import { LocalNotifications, ILocalNotification } from '@ionic-native/local-notifications/ngx';
const timeAt = new Date(this.notifyAt);
const hour = timeAt.getHours();
const minute = timeAt.getMinutes();
const title = 'Notify...';
const text = 'Hi, this is test notification.';
const notifications: ILocalNotification[] = Array(7).fill(0).map((_, idx) => {
return { title, text, trigger: { every: {weekday: idx + 1, hour, minute} }
};
});
this.localNotifications.schedule(notifications);
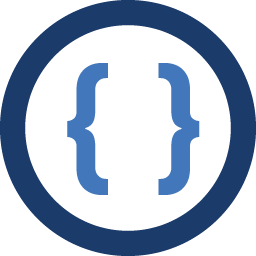
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am trying to implement reminder/alarm after a certain period of time in ionic2.I have found this plugin https://github.com/wnyc/cordova-plugin-wakeuptimer but I want to implement it using typescript as this is no recognizing window.wakeuptimer using in the below-mentioned code:
window.wakeuptimer.wakeup( successCallback, errorCallback, // a list of alarms to set { alarms : [{ type : 'onetime', time : { hour : 14, minute : 30 }, extra : { message : 'json containing app-specific information to be posted when alarm triggers' }, message : 'Alarm has expired!' }] } );
Can anyone help me out in this
-
Esco over 5 yearswhat if I want to trigger every day at the same time?
-
Khaled Ramadan about 5 yearsDid you figure it out? @Esco
-
Priyanka C over 4 years@Esco do you have any idea for trigger every day?
-
Volker Andres over 3 yearsGuys, just read the manual :D github.com/katzer/…