Remove an Element From HashTable?
Solution 1
That's because you're trying to remove the value, but the remove()
method expects a key.
When you're calling player.elements()
you get an enumeration of the values, not the keys. The remove()
method works on a key, not a value.
From HashTable remove() documentation
public V remove(Object key): Removes the key (and its corresponding value) from this hashtable. This method does nothing if the key is not in the hashtable.
Also, from the same documentation, here's a brief explanation on why it is not a good idea to use Enumeration
to remove elements:
Thus, in the face of concurrent modification, the iterator fails quickly and cleanly, rather than risking arbitrary, non-deterministic behavior at an undetermined time in the future. The Enumerations returned by Hashtable's keys and elements methods are not fail-fast.
What I would suggest you to do is to iterate over the entries, not the values.
for (Entry<Integer, String> entry: players.entrySet()){
String value = entry.getValue();
System.out.println(value);
if(value.startsWith("R")){
players.remove(entry.getKey());
System.out.println(value+"::Contains R");
}
}
This way you have both the key and the value in each iteration. You can examine the value
(which is the player's name) and then use the key
in order to manipulate your table.
Solution 2
for(Iterator<Map.Entry<Integer, String>> iter = players.entrySet().iterator(); iter.hasNext(); ) {
Map.Entry<Integer, String> e = iter.next();
if(e.getValue().startsWith("R")) {
iter.remove();
}
}
Solution 3
please add key
to remove()
method rather than value
.
Hashtable<String, Integer> h = new Hashtable<String, Integer>();
h.put("n1", 30);
h.put("n2", 30);
h.remove("n1"); //here we are giving "n1" which is key of the hashTable
Solution 4
You need to pass key
as argument in hashTable.remove()
to remove from HashTable
.
Reference : Remove from HashTable
Best way to remove an entry from a hash table. This may be helpful to you, have a look at it.
Solution 5
Don't remove from enumerations.
"Thus, in the face of concurrent modification, the iterator fails quickly and cleanly, rather than risking arbitrary, non-deterministic behavior at an undetermined time in the future. The Enumerations returned by Hashtable's keys and elements methods are not fail-fast."
You should using iterator:
import java.util.Hashtable;
import java.util.Iterator;
public class Test {
public static void main(String[] args) {
Hashtable<Integer,String> players = new Hashtable<Integer,String>();
players.put(1, "Sachin Tendulkar");
players.put(2, "Rahul Dravid");
players.put(3, "Virat Kohli");
players.put(4, "Rohit Sharma");
Iterator<Integer> iterators = players.keySet().iterator();
while(iterators.hasNext()) {
int key = iterators.next();
if(players.get(key).startsWith("R")) {
iterators.remove();
}
}
System.out.println(players);
}
}
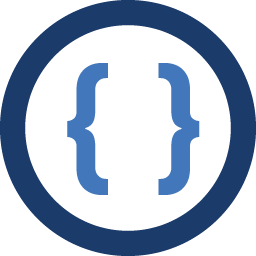
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I want to remove elements from
HashTable
, I usehashTable.remove()
for this but not gettingHashtable<Integer,String> players = new Hashtable<Integer,String>(); players.put(1, "Sachin Tendulkar"); players.put(2, "Rahul Dravid"); players.put(3, "Virat Kohli"); players.put(4, "Rohit Sharma"); Enumeration<String> enumration = players.elements(); while(enumration.hasMoreElements()){ String elmnt = enumration.nextElement(); System.out.println(elmnt); if(elmnt.startsWith("R")){ players.remove(elmnt); System.out.println(elmnt+"::Contains R"); } } System.out.println(players);
The output that i get is:
Rohit Sharma Rohit Sharma::Contains R Virat Kohli Rahul Dravid Rahul Dravid::Contains R Sachin Tendulkar {4=Rohit Sharma, 3=Virat Kohli, 2=Rahul Dravid, 1=Sachin Tendulkar}