Remove Elements from a HashSet while Iterating
Solution 1
You can manually iterate over the elements of the set:
Iterator<Integer> iterator = set.iterator();
while (iterator.hasNext()) {
Integer element = iterator.next();
if (element % 2 == 0) {
iterator.remove();
}
}
You will often see this pattern using a for
loop rather than a while
loop:
for (Iterator<Integer> i = set.iterator(); i.hasNext();) {
Integer element = i.next();
if (element % 2 == 0) {
i.remove();
}
}
As people have pointed out, using a for
loop is preferred because it keeps the iterator variable (i
in this case) confined to a smaller scope.
Solution 2
The reason you get a ConcurrentModificationException
is because an entry is removed via Set.remove() as opposed to Iterator.remove(). If an entry is removed via Set.remove() while an iteration is being done, you will get a ConcurrentModificationException. On the other hand, removal of entries via Iterator.remove() while iteration is supported in this case.
The new for loop is nice, but unfortunately it does not work in this case, because you can't use the Iterator reference.
If you need to remove an entry while iteration, you need to use the long form that uses the Iterator directly.
for (Iterator<Integer> it = set.iterator(); it.hasNext();) {
Integer element = it.next();
if (element % 2 == 0) {
it.remove();
}
}
Solution 3
Java 8 Collection has a nice method called removeIf that makes things easier and safer. From the API docs:
default boolean removeIf(Predicate<? super E> filter)
Removes all of the elements of this collection that satisfy the given predicate.
Errors or runtime exceptions thrown during iteration or by the predicate
are relayed to the caller.
Interesting note:
The default implementation traverses all elements of the collection using its iterator().
Each matching element is removed using Iterator.remove().
Solution 4
you can also refactor your solution removing the first loop:
Set<Integer> set = new HashSet<Integer>();
Collection<Integer> removeCandidates = new LinkedList<Integer>(set);
for(Integer element : set)
if(element % 2 == 0)
removeCandidates.add(element);
set.removeAll(removeCandidates);
Solution 5
Like timber said - "Java 8 Collection has a nice method called removeIf that makes things easier and safer"
Here is the code that solve your problem:
set.removeIf((Integer element) -> {
return (element % 2 == 0);
});
Now your set contains only odd values.
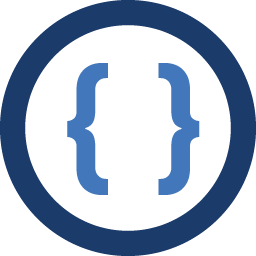
Admin
Updated on January 27, 2021Comments
-
Admin over 3 years
So, if I try to remove elements from a Java HashSet while iterating, I get a ConcurrentModificationException. What is the best way to remove a subset of the elements from a HashSet as in the following example?
Set<Integer> set = new HashSet<Integer>(); for(int i = 0; i < 10; i++) set.add(i); // Throws ConcurrentModificationException for(Integer element : set) if(element % 2 == 0) set.remove(element);
Here is a solution, but I don't think it's very elegant:
Set<Integer> set = new HashSet<Integer>(); Collection<Integer> removeCandidates = new LinkedList<Integer>(); for(int i = 0; i < 10; i++) set.add(i); for(Integer element : set) if(element % 2 == 0) removeCandidates.add(element); set.removeAll(removeCandidates);
Thanks!
-
Brett almost 15 yearsI prefer
for
towhile
, but each to his/her own. -
Adam Paynter almost 15 yearsI also use
for
myself. I usedwhile
to hopefully make the example clearer. -
Kathy Van Stone almost 15 yearsI perfer
for
mostly because the iterator variable is then limited to the scope of the loop. -
Steve Kuo almost 15 yearsIf
while
is used then the iterator's scope is larger than it needs to be. -
pepe450 over 14 yearsI prefer the while because it looks cleaner to me. The scope of the iterator should not be an issue if you are factoring your code. See Becks book "Test Driven Development" or Fowler's "Refactoring" for more about factoring code.
-
Romain F. over 10 yearsI would not recommend this as it introduces a hidden temporal coupling.
-
Bob Barbara over 8 yearsThat (or creating an
ArrayList
out of the set) is the best solution if you happen to not only remove existing elements but also adding new ones to the set during the loop. -
dustmachine about 8 yearsThis answer really starts showing its age... There's a Java-8 way of doing this now which is arguably cleaner.
-
Jelle den Burger over 7 yearsAn example:
integerSet.removeIf(integer-> integer.equals(5));
-
saurabheights about 7 years@RomainF. - What do you mean by hidden temporal coupling? Do you mean thread safe? Second, neither I would recommend this but the solution does have its pro. Super easy to read and hence maintainable.
-
saurabheights about 7 years@Shouldn't your code actually call it.next()?
-
Romain F. about 7 yearsYes, the for-loop produces a side effect, but I agree that it may be the most readable solution, unless you are using Java 8. Otherwise, just use "removeIf" method.
-
sjlee about 7 yearsThanks for that. Fixed.
-
KeithWM almost 7 yearsI think this answer misses the point that the first loop was only there to have a
HashSet
from which to remove certain elements. -
Phil Freihofner over 6 yearsAt what point is 'element' instantiated?
-
sjlee over 6 yearsUgh. Fixed. Thanks.
-
Sumit Kumar Saha over 5 yearswon't it throw ConcurrentModificationexception?
-
chaserb about 4 years@SumitKumarSaha, no, it shouldn't if you are modifying the collection with the iterator, as is done in both examples here. If another party has modified the collection while you're iterating, or if you modify it outside the iterator, the behavior is unspecified: javadoc
-
qwerty over 3 yearsIs there a better method to use, or were you just referring to the ability to use a lambda in place of an anonymous inner class?
-
dustmachine over 3 yearsHere's the more modern way:
myIntegerSet.stream().filter((it) -> it % 2 != 0).collect(Collectors.toSet())