Remove everything after space in string
Solution 1
strsplit("my string is sad"," ")[[1]][1]
Solution 2
You may use a regex like
sub(" .*", "", x)
See the regex demo.
Here, sub
will only perform a single search and replace operation, the .*
pattern will find the first space (since the regex engine is searching strings from left to right) and .*
matches any zero or more characters (in TRE regex flavor, even including line break chars, beware when using perl=TRUE
, then it is not the case) as many as possible, up to the string end.
Some variations:
sub("[[:space:]].*", "", x) # \s or [[:space:]] will match more whitespace chars
sub("(*UCP)(?s)\\s.*", "", x, perl=TRUE) # PCRE Unicode-aware regex
stringr::str_replace(x, "(?s) .*", "") # (?s) will force . to match any chars
See the online R demo.
Solution 3
or, substitute everything behind the first space to nothing:
gsub(' [A-z ]*', '' , 'my string is sad')
And with numbers:
gsub('([0-9]+) .*', '\\1', c('c123123123 0320.1'))
Solution 4
If you want to do it with a regex:
gsub('([A-z]+) .*', '\\1', 'my string is sad')
Solution 5
Stringr is your friend.
library(stringr)
word("my string is sad", 1)
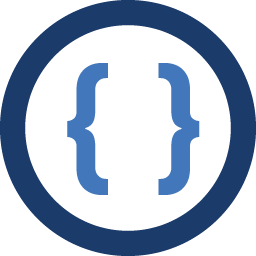
Admin
Updated on September 23, 2021Comments
-
Admin over 2 years
I would like to remove everything after a space in a string.
For example:
"my string is sad"
should return
"my"
I've been trying to figure out how to do this using sub/gsub but have been unsuccessful so far.
-
Monica Heddneck over 6 yearsa vectorized version to apply across a column of a dataframe would be even cooler
-
Monica Heddneck over 6 yearsWatch out, the top example can't remove a period!