Remove leading zeros from a number in a string
Solution 1
I hope this works:
try {
int i = Integer.parseInt(yourString);
System.out.println(i);
} catch(NumberFormatException e) {
// the string is not a number
}
Solution 2
There are many ways you might remove leading zeros from your String
; one approach, iterate the String
from left to right stopping at the next to the last character (so you don't erase the final digit) stop if you encounter a non-zero and invoke String.substring(int)
like
String str = "0000819780";
for (int i = 0, length = str.length() - 1; i < length; i++) {
if (str.charAt(i) != '0') {
str = str.substring(i);
break;
}
}
System.out.println(str);
Alternatively, parse the String
like
int v = Integer.parseInt(str);
System.out.println(v);
Solution 3
Alternative:
Using Apache Commons StringUtils class:
StringUtils.stripStart(String str, String stripChars);
For your example:
StringUtils.stripStart("0000819780", "0"); //should return "819780"
Solution 4
An elegant pure java way is just "0000819780".replaceFirst("^0+(?!$)", "")
Solution 5
If the input is always a number and you want to remove only the beginning zeroes, just parse it into an integer. If you want it in string format, parse it back.
String a = "0000819780";
System.out.println(Long.parseLong(a));
Check https://ideone.com/7NMxbE for a running code.
If it can have values other than numbers, you would need to catch NumberFormatException
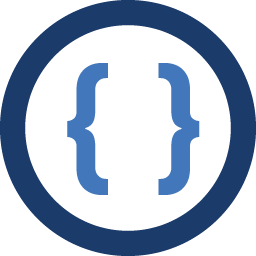
Admin
Updated on June 27, 2022Comments
-
Admin almost 2 years
I wanna trim this string.
"0000819780"
How i can get just the "819780" part.
I can do it with my way, just curious is there any "elegant" or "right" way to do this.