Remove specific properties from JSON object
30,242
You can parse the string first:
var temp = JArray.Parse(json);
temp.Descendants()
.OfType<JProperty>()
.Where(attr => attr.Name.StartsWith("_umb_"))
.ToList() // you should call ToList because you're about to changing the result, which is not possible if it is IEnumerable
.ForEach(attr => attr.Remove()); // removing unwanted attributes
json = temp.ToString(); // backing result to json
UPDATE OR:
result.Properties()
.Where(attr => attr.Name.StartsWith("_umb_"))
.ToList()
.ForEach(attr => attr.Remove());
UPDATE #2
You can specify more conditions in where
clause:
.Where(attr => attr.Name.StartsWith("_umb_") && some_other_condition)
OR
.Where(attr => attr.Name.StartsWith("_umb_") || some_other_condition)
Or whatever you need.
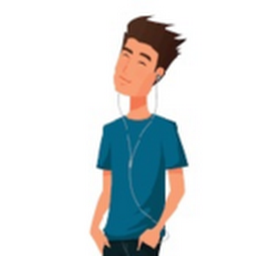
Author by
Harshit
Updated on January 23, 2022Comments
-
Harshit over 2 years
I have a JSON:
{ "scbs_currentstatus": "", "scbs_primaryissue": "", "_umb_id": "Test", "_umb_creator": "Admin", "_umb_createdate": "0001-01-01 00:00:00", "_umb_updatedate": "0001-01-01 00:00:00", "_umb_doctype": "Test", "_umb_login": "Test", "_umb_email": "Test", "_umb_password": { "newPassword": "Test", "oldPassword": null, "reset": null, "answer": null }, "_umb_membergroup": { " User": false, "Line User": true, "Callback User": false, "Su User": false, }, "umbracoMemberComments": "Test", "umbracoMemberFailedPasswordAttempts": "" }
Iam trying to remove all the properties start with "umb_" .is this possible in json.net?
and output will be like:
{ "scbs_currentstatus": "", "scbs_primaryissue": "", "umbracoMemberComments": "Test", "umbracoMemberFailedPasswordAttempts": "" }
using remove i am able to to do it however not all at a time.
result.Property("_umb_id").Remove();
any suggestion please?
-
Harshit about 8 years@javed. can we specify more than one condition in it?suppose we dont the fields contains "umbracoMember" in the property.