Rename a file/Image in flutter
Solution 1
First import the path package.
import 'package:path/path.dart' as path;
Then create a new target path to rename the file.
File picture = await ImagePicker.pickImage(
maxWidth: 800,
imageQuality: 10,
source: ImageSource.camera,
maxHeight: 800,
);
print('Original path: ${picture.path}');
String dir = path.dirname(picture.path);
String newPath = path.join(dir, 'case01wd03id01.jpg');
print('NewPath: ${newPath}');
picture.renameSync(newPath);
Solution 2
Use this function to rename filename only without change path of the file. you can use this function with or without image_picker.
import 'dart:io';
Future<File> changeFileNameOnly(File file, String newFileName) {
var path = file.path;
var lastSeparator = path.lastIndexOf(Platform.pathSeparator);
var newPath = path.substring(0, lastSeparator + 1) + newFileName;
return file.rename(newPath);
}
read more documentation in file.dart on your dart sdk. hope can help, regard
Solution 3
Manish Raj's answer isn't working for me since image_picker: ^0.6.7
, they recently changed their API when getting an image or video which returns PickedFile
instead of File
.
The new method I used was to convert from PickedFile
to File
then copy it over to applications directory with a new name. This method requires path_provider.dart
:
import 'package:path_provider/path_provider.dart';
...
...
PickedFile pickedFile = await _picker.getImage(source: ImageSource.camera);
// Save and Rename file to App directory
String dir = (await getApplicationDocumentsDirectory()).path;
String newPath = path.join(dir, 'case01wd03id01.jpg');
File f = await File(pickedF.path).copy(newPath);
I know the question states that they do not want to move it to a new folder but this was the best way I could find to make the rename work.
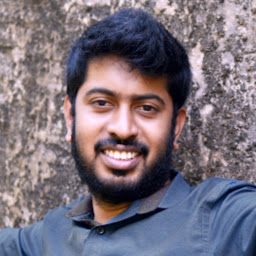
Comments
-
L Uttama over 1 year
I am picking a image from gallery/taking a photo using image_picker: ^0.6.2+3 package.
File picture = await ImagePicker.pickImage( maxWidth: 800, imageQuality: 10, source: source, // source can be either ImageSource.camera or ImageSource.gallery maxHeight: 800, );
and I get picture.path as
/Users/[some path]/tmp/image_picker_A0EBD0C1-EF3B-417F-9F8A-5DFBA889118C-18492-00001AD95CF914D3.jpg
Now I want to rename the image to case01wd03id01.jpg
Note : I don't want to move it to new folder
How can I rename it? I could not find it in official docs.
-
Akbar Pulatov about 2 yearsCannot rename file to '/var/mobile/Containers/Data/Application/66BC8D60-EF60-4DB2-A267-583A865D494E/Library/Caches/libCachedImageData/case01wd03id01.jpg', path = '/var/mobile/Containers/Data/Application/66BC8D60-EF60-4DB2-A267-583A865D494E/Library/Caches/libCachedImageData/23f98b30-8f13-11ec-bff1-03432be54c34.bin' (OS Error: No such file or directory, errno = 2)