Render dynamic HTML with embedded Razor variables using MVC
Solution 1
Use this line. I hope this may help.
@Html.Raw(System.Web.HttpUtility.HtmlDecode(@model.DisplayMessage))
EDIT 1
You can use any Razor Compiler like the one mentioned below
RazorEngine:
string result = RazorEngine.Razor.Parse(@model.DisplayMessage, new { Name = "Name" });
RazorEngine does not support any of the Mvc helpers such as Html and Url. Since these libraries are supposed to exist outside of Mvc and thus require more work to get them to work with those helpers.**
EDIT 2
You can use a Razor compiler that allows you to use HTML templates called RazorEngine which can be found at https://github.com/Antaris/RazorEngine
From Visual Studio, using the Package Manager Console command:
Install-Package RazorEngine
After installation I changed my controller as follows:
MessageController.cs
public ActionResult ShowMessage()
{
var htmlTemplate = _messageDAL.getHtmlMessage();
var htmlToDisplay = Engine.Razor.RunCompile(htmlTemplate , "messageTemplateKey", null, new { Name = "some model data" });
var messageVm = new MessageVm
{
DisplayMessage = htmlToDisplay;
};
return View("Index.cshtml", "", messageVm);
}
Solution 2
You can use a Razor compiler that allows you to use HTML templates called RazorEngine which can be found at https://github.com/Antaris/RazorEngine
From Visual Studio, using the Package Manager Console command:
Install-Package RazorEngine
After installation I changed my controller as follows:
MessageController.cs
public ActionResult ShowMessage()
{
var htmlTemplate = _messageDAL.getHtmlMessage();
var htmlToDisplay = Engine.Razor.RunCompile(htmlTemplate , "messageTemplateKey", null, new { Name = "some model data" });
var messageVm = new MessageVm
{
DisplayMessage = htmlToDisplay;
};
return View("Index.cshtml", "", messageVm);
}
And it worked first time. Big thanks to @Mukesh Kumar who provided the vital clues to rewrite the code which I've posted as a complete and working answer here.
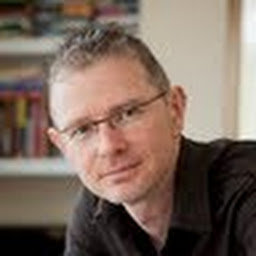
Jeff Yates
C#, .Net, MVC, Angular JS, SQL Server Full Stack Developer by Day Psychotherapist by night.
Updated on July 22, 2022Comments
-
Jeff Yates almost 2 years
I have some encoded Html which have any number of 1000s of different Razor variables embedded within it that I have stored and need to retrieve from the database. I want to be able to render this in a MVC/razor view.
Just one simple example of the html saved on the database (it can be more complex):
"<span>Your page is @Config.PageColour and you have page size of @Config.PageSize</span>"
MessageController.cs
public ActionResult ShowMessage() { var htmlToDisplay = _messageDAL.getHtmlMessage(); var messageVm = new MessageVm { DisplayMessage = htmlToDisplay; }; return View("Index.cshtml", "", messageVm); }
Index.cshtml
<html> @Html.Raw(@model.DisplayMessage) </html>
Results
When I run this the rendered page looks like this:
Your page is @Config.PageColour and you have page size of @Config.PageSize
But I want it to interpret the value of the Razor variable with the html block and should look like this:
Your page is Blue and you have page size of
A4
Really stuck on this so any help would be appreciated!