Replace last index of , with and in jQuery /JavaScript
13,918
Solution 1
lastIndexOf
finds the last index of the parameter string passed in it.
var x = 'a,b,c';
var pos = x.lastIndexOf(',');
x = x.substring(0,pos)+' and '+x.substring(pos+1);
console.log(x);
you can also use this function
function replace_last_comma_with_and(x) {
var pos = x.lastIndexOf(',');
return x.substring(0, pos) + ' and ' + x.substring(pos + 1);
}
console.log(replace_last_comma_with_and('a,b,c,d'));
An alternative solution using regex:
function replaceLastCommaWith(x, y) {
return x.replace(/,(?=[^,]*$)/, " " + y + " ");
}
console.log(replaceLastCommaWith("a,b,c,d", "and")); //a,b,c and d
console.log(replaceLastCommaWith("a,b,c,d", "or")); //a,b,c or d
Solution 2
This regex should do the job
"a,b,c,d".replace(/(.*),(.*)$/, "$1 and $2")
Solution 3
Try the following
var x= 'a,b,c,d';
x = x.replace(/,([^,]*)$/, " and $1");
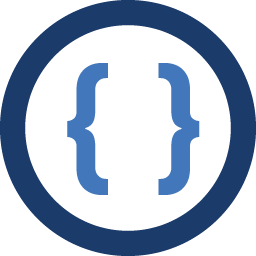
Author by
Admin
Updated on June 22, 2022Comments
-
Admin almost 2 years
i want to replace the last index of comma (
,
)in string withand
.eg .
a,b,c
with'a,b and c'
eg
q,w,e
withq,w and e