Request method 'GET' not supported Spring MVC
Solution 1
I'm going to start an answer and add to it as details become available. For your current error
org.springframework.web.bind.MissingServletRequestParameterException: Required String parameter 'selected' is not present
This happens because when you have something like
@RequestParam String selected,
And @RequestParam
doesn't have a value
attribute set, Spring will use the name of the parameter to look for the request parameter to bind. In your form, you obviously don't have a parameter named selected
. What you want is to get the value in
<select id="cJobs" name="cJobs" >
So change your @RequestParam
to
@RequestParam(value = "cJobs") String selected
to match the name
attribute of the select
input element.
Solution 2
Replace
@RequestMapping(value = MAIN_VIEW, method = RequestMethod.POST)
with
@RequestMapping(value = MAIN_VIEW, method = RequestMethod.GET)
You should also consider changing the form POST method. Your use case is more of Get rather than POST
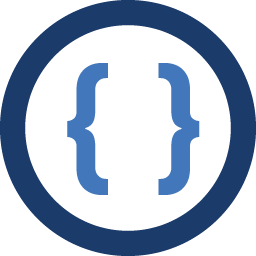
Admin
Updated on February 06, 2020Comments
-
Admin about 4 years
I have the following code in the controller
private static final String CJOB_MODEL = "cJobNms"; @RequestMapping(value = MAIN_VIEW, method = RequestMethod.POST) public String showTestXsd(//@ModelAttribute(FORM_MODEL) TestXsdForm xsdForm, //@ModelAttribute(MODEL) @Valid //TestXsdTable testXsdTable, @RequestParam String selected, Model model) throws DataException { String cJobNm =null; List<String> cJobNmList = null; System.out.println("selected:"+ selected); String xsdView = testXsdService.getXsdString(); cJobNmList = testXsdService.getCJobNm(); Set<String> cJobNmSet = new HashSet<String>(cJobNmList); TestXsdForm xsdForm = new TestXsdForm(); model.addAttribute("xsdView", xsdView); model.addAttribute("xsdFormModel", xsdForm); model.addAttribute(CJOB_MODEL, cJobNmSet); xsdForm.setXsdString(xsdView); return MAIN_VIEW; }
And the following code in my jsp.
<form:form modelAttribute="testXsdTable" name="xsdForm" action="/xsdtest/testXsdTables" id="xsdForm" method="POST" class="form" enctype="multipart/form-data" > <tr> <td> <label for="cJobs" class="fieldlabel">Jobs:</label> <select id="cJobs" name="cJobs" > <option value="${selected}" selected>${selected}</option> <c:forEach items="${cJobNms}" var="table"> <c:if test="${table != selected}"> <option value="${table}">${table}</option> </c:if> </c:forEach> </select> </td> </tr> <pre> <c:out value="${xsdForm.xsdString}"/> </pre> <div class="confirmbuttons"> <a href="#"class="button positive" id="saveXsdButton" onclick="saveTestXsd();"> <span class="confirm">Save</span> </a> </div>
When the user selects an option from the cJobNms list the selected value should be displayed in the controller method showTestXsd. Please let me know what I am doing wrong.
Currently I am getting a message : Request method 'GET' not supported
@RequestMapping(value = SAVE_VIEW, method = RequestMethod.POST) public String saveTestXsd( @ModelAttribute(MODEL) @Valid TestXsdTable testXsdTable, final BindingResult result, final Principal principal, Model model) throws DataException { boolean isNew = true; System.out.println("entering saveTestXsd in controller"); Map<String,Object> modelMap = model.asMap(); String xsdView = (String)modelMap.get("xsdView"); System.out.println("xsdView:::"+ xsdView); if(testXsdTable!= null){ System.out.println("xsdView(testXsdForm):::"+ testXsdTable.getXsdView()); } // Check for validation errors if (result.hasErrors()) { return SAVE_VIEW; } // Get the user information User loggedInUser = (User) ((Authentication) principal) .getPrincipal(); return SAVE_VIEW; }