requests.HTTPError uncaught after a requests.get() 404 response
Solution 1
Interpreter is your friend:
import requests
requests.get('google.com/admin')
# MissingSchema: Invalid URL u'google.com/admin': No schema supplied
Also, requests
exceptions:
import requests.exceptions
dir(requests.exceptions)
Also notice that by default requests
doesn't raise exception if status is not 200
:
In [9]: requests.get('https://google.com/admin')
Out[9]: <Response [503]>
There is raise_for_status() method that does it:
In [10]: resp = requests.get('https://google.com/admin')
In [11]: resp
Out[11]: <Response [503]>
In [12]: resp.raise_for_status()
...
HTTPError: 503 Server Error: Service Unavailable
Solution 2
Running your code in python 2.7.5:
import requests
try:
response = requests.get('google.com/admin') #Should return 404
except requests.HTTPError, e:
print 'HTTP ERROR %s occured' % e.code
print e
Results in:
File "C:\Python27\lib\site-packages\requests\models.py", line 291, in prepare_url
raise MissingSchema("Invalid URL %r: No schema supplied" % url)
requests.exceptions.MissingSchema: Invalid URL u'google.com/admin': No schema supplied
To get your code to pick up this exception you need to add:
except (requests.exceptions.MissingSchema) as e:
print 'Missing schema occured. status'
print e
Note also it is not a missing schema but a missing scheme.
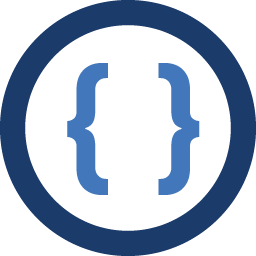
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
I'm having a slight problem with the requests library.
Say for example I have a statement like this in Python:
try: request = requests.get('google.com/admin') #Should return 404 except requests.HTTPError, e: print 'HTTP ERROR %s occured' % e.code
For some reason the exception is not being caught. I've checked the API documentation for requests but it's a bit slim. Is there anyone who has more experience with the library that might be able to help me out?