Required request body content is missing: org.springframework.web.method.HandlerMethod$HandlerMethodParameter
Solution 1
Sorry guys.. actually because of a csrf token was needed I was getting that issue. I have implemented spring security and csrf is enable. And through ajax call I need to pass the csrf token.
Solution 2
You shouldn't send a request body with an HTTP GET request. You should modify addDepartment()
so that it only supports POST, and POST your JSON to that endpoint. If you want to GET information about a department, you should create a separate controller method that does that (and does not require a request body).
Also, double-check your endpoint definitions since you have misspelled "reimbursement" in the $.ajax
call.
Solution 3
I also had the same problem. I use "Postman" for JSON request. The code itself is not wrong. I simply set the content type to JSON (application/json
) and it worked, as you can see on the image below
Solution 4
Try this:
@RequestBody(required = false) String str
Solution 5
I made some minor modification to you code and tested it with a spring project that I have and it works, The logic will only work with POST if I use GET it throws an error with invalid request. Also in your ajax call I commented out commit(true), the browser debugger flagged and error that it is not defined. Just modify the url to fit your Spring project architecture.
$.ajax({
url: "/addDepartment",
method: 'POST',
dataType: 'json',
data: "{\"message\":\"abc\",\"success\":true}",
contentType: 'application/json',
mimeType: 'application/json',
success: function(data) {
alert(data.success + " " + data.message);
//commit(true);
},
error:function(data,status,er) {
alert("error: "+data+" status: "+status+" er:"+er);
}
});
@RequestMapping(value="/addDepartment", method=RequestMethod.POST)
public AjaxResponse addDepartment(@RequestBody final AjaxResponse departmentDTO)
{
System.out.println("addDepartment: >>>>>>> "+departmentDTO);
AjaxResponse response=new AjaxResponse();
response.setSuccess(departmentDTO.isSuccess());
response.setMessage(departmentDTO.getMessage());
return response;
}
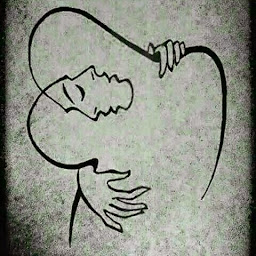
chaitanya dalvi
Updated on November 16, 2020Comments
-
chaitanya dalvi over 3 years
Error to Pass JSON data from JSP to controller in ResponseBody.
07:13:53.919 DEBUG o.s.w.s.m.m.a.ExceptionHandlerExceptionResolver - Resolving exception from handler [public com.chaitanya.ajax.AjaxResponse com.chaitanya.web.controller.DepartmentController.addDepartment(com.chaitanya.ajax.AjaxResponse)]: org.springframework.http.converter.HttpMessageNotReadableException: Required request body content is missing: org.springframework.web.method.HandlerMethod$HandlerMethodParameter@98d8d36c 07:13:54.106 DEBUG o.s.w.s.m.a.ResponseStatusExceptionResolver - Resolving exception from handler [public com.chaitanya.ajax.AjaxResponse com.chaitanya.web.controller.DepartmentController.addDepartment(com.chaitanya.ajax.AjaxResponse)]: org.springframework.http.converter.HttpMessageNotReadableException: Required request body content is missing: org.springframework.web.method.HandlerMethod$HandlerMethodParameter@98d8d36c 07:13:54.125 DEBUG o.s.w.s.m.s.DefaultHandlerExceptionResolver - Resolving exception from handler [public com.chaitanya.ajax.AjaxResponse com.chaitanya.web.controller.DepartmentController.addDepartment(com.chaitanya.ajax.AjaxResponse)]: org.springframework.http.converter.HttpMessageNotReadableException: Required request body content is missing: org.springframework.web.method.HandlerMethod$HandlerMethodParameter@98d8d36c 07:1
Ajax Call:
$.ajax({ url: "/BusinessReimbursment/addDepartment", method: 'POST', dataType: 'json', data: "{\"message\":\"abc\",\"success\":true}", contentType: 'application/json', mimeType: 'application/json', success: function(data) { alert(data.id + " " + data.name); commit(true); }, error:function(data,status,er) { alert("error: "+data+" status: "+status+" er:"+er); } });
Controller:
@RestController public class DepartmentController { @Autowired @Qualifier("departmentService") private DepartmentService departmentService; @RequestMapping(value="/addDepartment", method={RequestMethod.POST}) public @ResponseBody AjaxResponse addDepartment(@RequestBody AjaxResponse departmentDTO){ AjaxResponse response=new AjaxResponse(); return response; }
AppConfig.java
@Bean
public RequestMappingHandlerAdapter annotationMethodHandlerAdapter() { final RequestMappingHandlerAdapter annotationMethodHandlerAdapter = new RequestMappingHandlerAdapter(); final MappingJackson2HttpMessageConverter mappingJacksonHttpMessageConverter = new MappingJackson2HttpMessageConverter(); List<HttpMessageConverter<?>> httpMessageConverter = new ArrayList<HttpMessageConverter<?>>(); httpMessageConverter.add(mappingJacksonHttpMessageConverter); String[] supportedHttpMethods = { "POST", "GET", "HEAD" }; annotationMethodHandlerAdapter.setMessageConverters(httpMessageConverter); annotationMethodHandlerAdapter.setSupportedMethods(supportedHttpMethods); return annotationMethodHandlerAdapter; }
please help me to get out out of that. I m using Spring 4, jakson 2.3.0
If i try to POST request it gives:org.springframework.web.HttpRequestMethodNotSupportedException: Request method 'POST' not supported
-
chaitanya dalvi about 9 yearsI hv mention GET n POST in request mapping annotation. If I pass post response then it gives error which I hv mention in post..
-
ach about 9 yearsPlease read my answer again. You can't use
GET
in your@RequestMapping
annotation along with@RequestBody
since a GET request cannot have a body. In general GET should only be used for read-only operations like getting a record or searching. Remove GET completely and try again. -
chaitanya dalvi about 9 yearsI changed Method: POST, and Removed RequestMethod:GET From @requestMapping annotion i get following error which i hv mention in post.
-
chaitanya dalvi about 9 years
Request method 'POST' not supported Invalid CSRF token found for http://localhost:8080/BusinessReimbursment/addDepartment DispatcherServlet with name 'dispatcher' processing POST request for [/BusinessReimbursment/403] Looking up handler method for path /403 HttpRequestMethodNotSupportedException: Request method 'POST' not supported HttpRequestMethodNotSupportedException: Request method 'POST' not supported HttpRequestMethodNotSupportedException: Request method 'POST' not supported WARN o.s.web.servlet.PageNotFound - Request method 'POST' not supported
-
chaitanya dalvi about 9 years
Request method 'POST' not supported Invalid CSRF token found for http://localhost:8080/BusinessReimbursment/addDepartment DispatcherServlet with name 'dispatcher' processing POST request for [/BusinessReimbursment/403] Looking up handler method for path /403 HttpRequestMethodNotSupportedException: Request method 'POST' not supported HttpRequestMethodNotSupportedException: Request method 'POST' not supported HttpRequestMethodNotSupportedException: Request method 'POST' not supported WARN o.s.web.servlet.PageNotFound - Request method 'POST' not supported –
-
csvan over 7 years
Invalid CSRF token
is a completely different problem. -
default locale almost 7 yearsIs this a question? If so, post it as a separate question. You can add a link to this question, if necessary.
-
default locale almost 7 yearsNext time, don't turn your whole post into a link, better add a link at the end. Also, OP didn't say anything about Postman. If you want to address this issue it seems like a good idea to show what was the particular mistake and how to reproduce the error.
-
Alexander almost 6 yearsNo, this will probably not help
-
JackTheKnife about 5 yearsSince when you can't send a request body with an HTTP GET request? More here: stackoverflow.com/questions/978061/http-get-with-request-body
-
ach about 5 yearsI was incorrect about that in my answer from 4 years ago. If you'd like to submit an edit to change the word "can't" to "shouldn't", feel free.
-
Zhou over 4 years@ach Actually you're right to some extent. The RestTemplate of the Spring framework does throw the message body away if the request is GET even if the HTTP spec no longer disallows this. I clearly saw it in its source code.
-
AylaWinters about 3 yearsI don't understand why this worked, but it did and my requestbody went through were before it did not...
-
Harsh Gundecha almost 3 years@Juan this is same as not using annotation at all and it would still work, not a solution