Rest Assured Exception when test fails
Solution 1
Yes, it will fail by throwing AssertionException
. You have two ways to avoid this:
- Catch the exception with
AssertionError
. Remove
expect().statusCode().body()
part and assign response to Response object and continue with desired tests by parsing it usingJsonPath
object as below.Response res = given(). param("thingAccountIsfor","ABCD"). when(). get("http://machine:2343/rest/accounts/getaccount"); JsonPath jp = new JsonPath(res.asString()); String account = jp.get("account").toString();
Solution 2
Response response = given().contentType("application/json")
param("thingAccountIsfor","ABCD").
.get("url")
.then()
.assertThat()
.statusCode(200).and()
.body("$", notNullValue())
.extract().response();
final JSONObject content = new JSONObject(response.getBody().asString());
String val=content.getString("account");
cleaner option
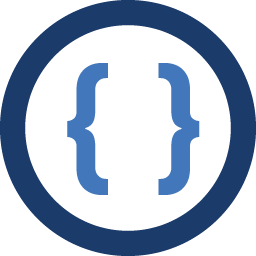
Admin
Updated on January 31, 2020Comments
-
Admin over 4 years
I have a simple rest-assured test that is verifying this json comes be with the correct data for a call. I am using rest-assured 1.8 and did swap the groovy jars for the groovy-all jar due to a known issue with 2 different versions of ASM.
My json the comes back looks like this:
{"account":"12345"}
My code looks like this:
package com.blah.tests; import org.junit.Test; import static com.jayway.restassured.RestAssured.given; import static org.hamcrest.Matchers.equalTo; public class AccountsTest { public AccountsTest() { } @Test public void getLocationAccount() { given(). param("thingAccountIsfor","ABCD"). expect(). statusCode(200). body("account",equalTo("10")). when(). get("http://machine:2343/rest/accounts/getaccount"); } }
When I run this matching the value i expect for the account it works fine. When I put in a value that does not match I get a stack trace:
log4j:WARN No appenders could be found for logger (org.apache.http.impl.conn.SingleClientConnManager). log4j:WARN Please initialize the log4j system properly. java.lang.AssertionError: 1 expectation failed. JSON path account doesn't match. Expected: 10 Actual: 12345 at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:39) at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:27) at java.lang.reflect.Constructor.newInstance(Constructor.java:513) at org.codehaus.groovy.reflection.CachedConstructor.invoke(CachedConstructor.java:77) at org.codehaus.groovy.reflection.CachedConstructor.doConstructorInvoke(CachedConstructor.java:71) at org.codehaus.groovy.runtime.callsite.ConstructorSite$ConstructorSiteNoUnwrap.callConstructor(ConstructorSite.java:81) at org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCallConstructor(CallSiteArray.java:57) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callConstructor(AbstractCallSite.java:182) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callConstructor(AbstractCallSite.java:190) at com.jayway.restassured.internal.ResponseSpecificationImpl$HamcrestAssertionClosure.validate(ResponseSpecificationImpl.groovy:399) at com.jayway.restassured.internal.ResponseSpecificationImpl$HamcrestAssertionClosure$validate.call(Unknown Source) at org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCall(CallSiteArray.java:45) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:108) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:116) at com.jayway.restassured.internal.RequestSpecificationImpl.invokeFilterChain(RequestSpecificationImpl.groovy:759) at com.jayway.restassured.internal.RequestSpecificationImpl$invokeFilterChain.callCurrent(Unknown Source) at org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCallCurrent(CallSiteArray.java:49) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callCurrent(AbstractCallSite.java:133) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callCurrent(AbstractCallSite.java:149) at com.jayway.restassured.internal.RequestSpecificationImpl.applyPathParamsAndSendRequest(RequestSpecificationImpl.groovy:1142) at com.jayway.restassured.internal.RequestSpecificationImpl.this$2$applyPathParamsAndSendRequest(RequestSpecificationImpl.groovy) at com.jayway.restassured.internal.RequestSpecificationImpl$this$2$applyPathParamsAndSendRequest.callCurrent(Unknown Source) at org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCallCurrent(CallSiteArray.java:49) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callCurrent(AbstractCallSite.java:133) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.callCurrent(AbstractCallSite.java:149) at com.jayway.restassured.internal.RequestSpecificationImpl.get(RequestSpecificationImpl.groovy:131) at com.jayway.restassured.specification.RequestSender$get.call(Unknown Source) at org.codehaus.groovy.runtime.callsite.CallSiteArray.defaultCall(CallSiteArray.java:45) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:108) at org.codehaus.groovy.runtime.callsite.AbstractCallSite.call(AbstractCallSite.java:120) at com.jayway.restassured.internal.ResponseSpecificationImpl.get(ResponseSpecificationImpl.groovy:226) at com.tim.tests.AccountsTest.getLocationAccount(AccountsTest.java:17) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:25) at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:45) at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:15) at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:42) at org.junit.internal.runners.statements.InvokeMethod.evaluate(InvokeMethod.java:20) at org.junit.runners.ParentRunner.runLeaf(ParentRunner.java:263) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:68) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:47) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:231) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:60) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:229) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:50) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:222) at org.junit.runners.ParentRunner.run(ParentRunner.java:300) at org.junit.runner.JUnitCore.run(JUnitCore.java:157) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:77) at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:195) at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:63) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:39) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:120) Process finished with exit code 255
I am running this inside Intellij. I did try to move the groovy jars, back in, move the conflicting version of the ASM off of my class path and re-run it. I got the same result so I don't beleive this has to do with using the groovy-all jar instead of the groovy jars that ship with rest-assured 1.8.
Does rest-assured throw an exception every time it has a test fail or is something wrong here?